24 Aug 2023
In this series we introduce the OpenRISC
glibc FPU
port and the effort required to get user space FPU support into OpenRISC Linux.
Adding FPU support to user space
applications is a full stack project covering:
Have a look at previous articles if you need to catch up. In this article we will cover
updates done to the Linux kernel, GCC (done long ago) and GDB for debugging.
Porting Linux to an FPU
Supporting hardware floating point operations in Linux user space applications
means adding the ability for the Linux kernel to store and restore FPU state
upon context switches. This allows multiple programs to use the FPU at the same
time as if each program has it’s own floating point hardware. The kernel allows
programs to multiplex usage of the FPU transparently. This is similar to how
the kernel allows user programs to share other hardware like the CPU and
Memory.
On OpenRISC this requires to only add one addition register, the floating point
control and status register (FPCSR
) to to context switches. The FPCSR
contains status bits pertaining to rounding mode and exceptions.
We will cover three places where Linux needs to store FPU state:
- Context Switching
- Exception Context (Signal Frames)
- Register Sets
Context Switching
In order for the kernel to be able to support FPU usage in user space programs
it needs to be able to save and restore the FPU state during context switches.
Let’s look at the different kinds of context switches that happen in the Linux
Kernel to understand where FPU state needs to be stored and restored.
For our discussion purposes we will define a context switch as being
when one CPU state (context) is saved from CPU hardware (registers and program
counter) to memory and then another CPU state is loaded form memory to CPU
hardware. This can happen in a few ways.
- When handling exceptions such as interrupts
- When the scheduler wants to swap out one process for another.
Furthermore, exceptions may be categorized into one of two cases: Interrupts and
System calls. For each of these a different amount of CPU state needs to be saved.
- Interrupts - for timers, hardware interrupts, illegal instructions etc,
for this case the full context will be saved. As the switch is not
anticipated by the currently running program.
- System calls - for system calls the user space program knows that it is making a function
call and therefore will save required state as per OpenRISC calling conventions.
In this case the kernel needs to save only callee saved registers.
Below are outlines of the sequence of operations that take place when
transitioning from Interrupts and System calls into kernel code. It highlights
at what point state is saved with the INT,
kINT and FPU labels.
The monospace
labels below correspond to the actual assembly labels in entry.S,
the part of the OpenRISC Linux kernel that handles entry into kernel code.
Interrupts (slow path)
EXCEPTION_ENTRY
- all INT state is saved
- Handle exception in kernel code
_resume_userspace
- Check thread_info
for work pending
- If work pending
_work_pending
- Call do_work_pending
- Check if reschedule needed
- If so, performs
_switch
which save/restores kINT
and FPU state
- Check for pending signals
- If so, performs
do_signal
which save/restores FPU state
RESTORE_ALL
- all INT state is restored and return to user space
System calls (fast path)
_sys_call_handler
- callee saved registers are saved
- Handle syscall in kernel code
_syscall_check_work
- Check thread_info
for work pending
- If work pending
- Save additional INT state
_work_pending
- Call do_work_pending
- Check if reschedule needed
- If so, performs
_switch
which save/restores kINT
and FPU state
- Check for pending signals
- If so, performs
do_signal
which save/restores FPU state
RESTORE_ALL
- all INT state is restored and return to user space
_syscall_resume_userspace
- restore callee saved registers return to user space.
Some key points to note on the above:
- INT denotes the interrupts program’s register state.
- kINT denotes the kernel’s register state before/after a context switch.
- FPU deones FPU state
- In both cases step 4 checks for work pending, which may cause task
rescheduling in which case a Context Switch (or task switch) will
be performed.
If rescheduling is not performed then after the sequence is complete processing
will resume where it left off.
- Step 1, when switching from user mode to kernel mode is called a Mode Switch
- Interrupts may happen in user mode or kernel mode
- System calls will only happen in user mode
- FPU state only needs to be saved and restored for user mode programs,
because kernel mode programs, in general, do not use the FPU.
- The current version of the OpenRISC port as of
v6.8
save and restores both
INT and FPU state
what is shown before is a more optimized mechanism of only saving FPU state when needed. Further optimizations could
be still make to only save FPU state for user space, and not save/restore if it is already done.
With these principal’s in mind we can now look at how the mechanics of context
switching works.
Upon a Mode Switch from user mode to kernel mode the process thread
stack switches from using a user space stack to the associated kernel space
stack. The required state is stored to a stack frame in a pt_regs
structure.
The pt_regs
structure (originally ptrace registers) represents the CPU
registers and program counter context that needs to be saved.
Below we can see how the kernel stack and user space stack relate.
kernel space
+--------------+ +--------------+
| Kernel Stack | | Kernel stack |
| | | | | |
| v | | | |
| pt_regs' -------\ | v |
| v | | | pt_regs' --------\
| pt_reg'' |<+ | | v |<+ |
| | | | | | | |
+--------------+ | | +--------------+ | |
| thread_info | | | | thread_info | | |
| *task | | | | *task | | |
| ksp ----------/ | | ksp-----------/ |
+--------------+ | +--------------+ |
| |
0xc0000000 | |
---------------------|----------------------|-----
| |
process a | process b |
+--------------+ | +------------+ |
| User Stack | | | User Stack | |
| | | | | | | |
| V |<---+ | v |<-----+
| | | |
| | | |
| text | | text |
| heap | | heap |
+--------------+ +------------+
0x00000000
user space
process a
In the above diagram notice how there are 2 set’s of pt_regs
for process a.
The pt_regs’ structure represents the user space registers (INT)
that are saved during the switch from user mode to kernel mode. Notice how
the pt_regs’ structure has an arrow pointing the user space stack, that’s the
saved stack pointer. The second pt_regs’’ structure represents the frozen
kernel state (kINT)
that was saved before a task switch was performed.
process b
Also in the diagram above we can see process b has only a pt_regs’ (INT)
structure saved on the stack and does not currently have a pt_regs’’ (kINT)
structure saved. This indicates that that this process is currently running in
kernel space and is not yet frozen.
As we can see here, for OpenRISC there are two places to store state.
- The mode switch context is saved to a
pt_regs
structure on the kernel
stack represented by pt_regs’ at this point only integer registers need to
be saved. This is represents the user process state.
- The context switch context is stored by OpenRISC again on the stack,
represented by pt_regs’‘. This represents the kernel’s state before a
task switch. All state that the kernel needs to resume later is stored. In
other architectures this state is not stored on the stack but to the
task
structure or to the thread_info
structure. This context may store the all
extra registers including FPU and Vector registers.
Note In the above diagram we can see the kernel stack and
thread_info
live in
the same memory space. This is a source of security issues and many
architectures have moved to support
virtually mapped kernel stacks,
OpenRISC does not yet support this and it would be a good opportunity
for improvement.
The structure of the pt_regs
used by OpenRISC is as per below:
struct pt_regs {
long gpr[32];
long pc;
/* For restarting system calls:
* Set to syscall number for syscall exceptions,
* -1 for all other exceptions.
*/
long orig_gpr11; /* For restarting system calls */
long dummy; /* Cheap alignment fix */
long dummy2; /* Cheap alignment fix */
};
The structure of thread_struct
now used by OpenRISC to store the
user specific FPU state is as per below:
struct thread_struct {
long fpcsr; /* Floating point control status register. */
};
The patches to OpenRISC added to support saving and restoring FPU state
during context switches are below:
- 2023-04-26 63d7f9f11e5e Stafford Horne openrisc: Support storing and restoring fpu state
- 2024-03-14 ead6248f25e1 Stafford Horne openrisc: Move FPU state out of pt_regs
Signals and Signal Frames.
Signal frames are another place that we want FPU’s state, namely FPCSR
, to be available.
When a user process receives a signal it executes a signal handler in the
process space on a stack slightly outside it’s current stack. This is setup
with setup_rt_frame.
As we saw above signals are received after syscalls or exceptions, during the
do_pending_work
phase of the entry code. This means means FPU state will need
to be saved and restored.
Again, we can look at the stack frames to paint a picture of how this works.
kernel space
+--------------+
| Kernel Stack |
| | |
| v |
| pt_regs' --------------------------\
| |<+ |
| | | |
| | | |
+--------------+ | |
| thread_info | | |
| *task | | |
| ksp ----------/ |
+--------------+ |
|
0xc0000000 |
------------------- |
|
process a |
+--------------+ |
| User Stack | |
| | | |
| V | |
|xxxxxxxxxxxxxx|> STACK_FRAME_OVERHEAD |
| siginfo |\ |
| ucontext | >- sigframe |
| retcode[] |/ |
| |<-----------------------/
| |
| text |
| heap |
+--------------+
0x00000000
user space
Here we can see that when we enter a signal handler, we can get a bunch of stuff
stuffed in the stack in a sigframe
structure. This includes the ucontext
,
or user context which points to the original state of the program, registers and
all. It also includes a bit of code, retcode
, which is a trampoline to bring us
back into the kernel after the signal handler finishes.
Note we could also setup an alternate
signalstack
to use instead of stuffing stuff onto the main user stack. The
above example is the default behaviour.
The user pt_regs
(as we called pt_regs’) is updated before returning to user
space to execute the signal handler code by updating the registers as follows:
sp: stack pinter updated to point to a new user stack area below sigframe
pc: program counter: sa_handler(
r3: argument 1: signo,
r4: argument 2: &siginfo
r5: argument 3: &ucontext)
r9: link register: retcode[]
Now, when we return from the kernel to user space, user space will resume in the
signal handler, which runs within the user process context.
After the signal handler completes it will execute the retcode
block which is setup to call the special system call rt_sigreturn.
Note for OpenRISC this means the stack has to be executable. Which is
a
major security vulnerability.
Modern architectures do not have executable stacks and use
vdso
or is provided by libc in
sa_restorer
.
The rt_sigreturn
system call will restore the ucontext
registers (which
may have been updated by the signal handler) to the user pt_regs
on the
kernel stack. This allows us to either restore the user context before the
signal was received or return to a new context setup by the signal handler.
A note on user space ABI compatibility for signals.
We need to to provide and restore the FPU FPCSR
during signals via
ucontext
but also not break user space ABI. The ABI is important because
kernel and user space programs may be built at different times. This means the
layout of existing fields in ucontext
cannot change. As we can see below by
comparing the ucontext
definitions from Linux, glibc and musl each program
maintains their own separate header file.
In Linux we cannot add fields to uc_sigcontext
as it would make uc_sigmask
unable to be read. Fortunately we had a bit of space in sigcontext
in the
unused oldmask
field which we could repurpose for FPCSR
.
The structure used by Linux to populate the signal frame is:
From: uapi/asm-generic/ucontext.h
struct ucontext {
unsigned long uc_flags;
struct ucontext *uc_link;
stack_t uc_stack;
struct sigcontext uc_mcontext;
sigset_t uc_sigmask;
};
From: uapi/asm/ptrace.h
struct sigcontext {
struct user_regs_struct regs; /* needs to be first */
union {
unsigned long fpcsr;
unsigned long oldmask; /* unused */
};
};
Note In
sigcontext
originally a
union
was not used
and
caused ABI breakage; which was soon fixed.
From: uapi/asm/sigcontext.h
struct user_regs_struct {
unsigned long gpr[32];
unsigned long pc;
unsigned long sr;
};
The structure that glibc expects is.
/* Context to describe whole processor state. */
typedef struct
{
unsigned long int __gprs[__NGREG];
unsigned long int __pc;
unsigned long int __sr;
} mcontext_t;
/* Userlevel context. */
typedef struct ucontext_t
{
unsigned long int __uc_flags;
struct ucontext_t *uc_link;
stack_t uc_stack;
mcontext_t uc_mcontext;
sigset_t uc_sigmask;
} ucontext_t;
Note This is broken, the struct mcontext_t
in glibc is
missing the space for oldmask
.
The structure used by musl is:
typedef struct sigcontext {
struct {
unsigned long gpr[32];
unsigned long pc;
unsigned long sr;
} regs;
unsigned long oldmask;
} mcontext_t;
typedef struct __ucontext {
unsigned long uc_flags;
struct __ucontext *uc_link;
stack_t uc_stack;
mcontext_t uc_mcontext;
sigset_t uc_sigmask;
} ucontext_t;
Below were the patches the to OpenRISC kernel to add floating point state to the
signal API. This originally caused some ABI breakage and was fixed in the second patch.
- 2023-04-26 27267655c531 Stafford Horne openrisc: Support floating point user api
- 2023-07-10 dceaafd66881 Stafford Horne openrisc: Union fpcsr and oldmask in sigcontext to unbreak userspace ABI
Register sets
Register sets provide debuggers the ability to read and save the state of
registers in other processes. This is done via the ptrace
PTRACE_GETREGSET
and PTRACE_SETREGSET
requests.
Regsets also define what is dumped to core dumps when a process crashes.
In OpenRISC we added the ability to get and set the FPCSR
register
with the following patches:
- 2023-04-26 c91b4a07655d Stafford Horne openrisc: Add floating point regset (shorne/or1k-6.4-updates, or1k-6.4-updates)
- 2024-03-14 14f89b18c117 Stafford Horne openrisc: Move FPU state out of pt_regs
Porting GCC to an FPU
Supporting FPU Instructions
I ported GCC to the OpenRISC FPU back in 2019
, this entailed defining new instructions in the RTL machine description for
example:
(define_insn "plussf3"
[(set (match_operand:SF 0 "register_operand" "=r")
(plus:SF (match_operand:SF 1 "register_operand" "r")
(match_operand:SF 2 "register_operand" "r")))]
"TARGET_HARD_FLOAT"
"lf.add.s\t%d0, %d1, %d2"
[(set_attr "type" "fpu")])
(define_insn "minussf3"
[(set (match_operand:SF 0 "register_operand" "=r")
(minus:SF (match_operand:SF 1 "register_operand" "r")
(match_operand:SF 2 "register_operand" "r")))]
"TARGET_HARD_FLOAT"
"lf.sub.s\t%d0, %d1, %d2"
[(set_attr "type" "fpu")])
The above is a simplified example of GCC Register Transfer Language(RTL)
lisp expressions. Note, the real expression actually uses mode iterators and is a bit harder to understand, hence the simplified version above.
These expressions are used for translating the GCC compiler RTL from it’s abstract syntax tree
form to actual machine instructions.
Notice how the above expressions are in the format (define_insn INSN_NAME RTL_PATTERN CONDITION MACHINE_INSN ...)
. If
we break it down we see:
INSN_NAME
- this is a unique name given to the instruction.
RTL_PATTERN
- this is a pattern we look for in the RTL tree, Notice how the lisp represents 3 registers connected by the instruction node.
CONDITION
- this is used to enable the instruction, in our case we use TARGET_HARD_FLOAT
. This means if the GCC hardware floating point
option is enabled this expression will be enabled.
MACHINE_INSN
- this represents the actual OpenRISC assembly instruction that will be output.
Supporting Glibc Math
In order for glibc to properly support floating point operations GCC needs to do
a bit more than just support outputting floating point instructions. Another
component of GCC is software floating point emulation. When there are
operations not supported by hardware GCC needs to fallback to using software
emulation. With way GCC and GLIBC weave software math routines and floating
point instructions we can think of the FPU as a math accelerator. For example,
the floating point square root operation is not provided by OpenRISC hardware.
When operations like square root are not available by hardware glibc will inject
software routines to handle the operation. The outputted square root routine
may use hardware multiply lf.mul.s
and divide lf.div.s
operations to
accelerate the emulation.
In order for this to work correctly the rounding mode and exception state of the
FPU and libgcc emulation need to by in sync. Notably, we had one patch to fix an
issue with exceptions not being in sync which was found when running glibc
tests.
- 2023-03-19 33fb1625992 or1k: Do not clear existing FPU exceptions before updating
The libc math routines include:
- Trigonometry functions - For example
float sinf (float x)
- Logarithm functions - For example
float logf (float x)
- Hyperbolic functions - For example
float ccoshf (complex float z)
The above just names a few, but as you can imagine the floating point acceleration
provided by the FPU
is essential for performant scientific applications.
Adding debugging capabilities for an FPU
FPU debugging allows a user to inspect the FPU specific registers.
This includes FPU state registers and flags as well as view the floating point
values in each general purpose register. This is not yet implemented on
OpenRISC.
This will be something others can take up. The work required is to map
Linux FPU register sets to GDB.
Summary
In summary adding floating point support to Linux revolved around adding one more register, the FPCSR
,
to context switches and a few other places.
GCC fixes were needed to make sure hardware and software floating point routines could work together.
There are still improvements that can be done for the Linux port as noted above. In the next
article we will wrap things up by showing the glibc port.
Further Reading
22 Aug 2023
In the last article we introduced the
OpenRISC glibc FPU port and the effort required to get user space FPU support
into OpenRISC linux user applications. We explained how the FPU port is a
fullstack project covering:
- Architecture Specification
- Simulators and CPU implementations
- Linux Kernel support
- GCC Instructions and Soft FPU
- Binutils/GDB Debugging Support
- glibc support
In this entry we will cover updating Simulators and CPU implementations to support
the architecture changes which are called for as per the previous article.
- Allowing usermode programs to update the FPCSR register
- Detecting tininess before rounding
Simulator Updates
The simulators used for testing OpenRISC software without hardware are QEMU
and or1ksim. They both needed to be updated to cohere to the specification
updates discussed above.
Or1ksim Updates
The OpenRISC architectue simulator or1ksim has been updated with the single patch:
cpu: Allow FPCSR to be read/written in user mode.
The softfloat FPU implementation was already configured to detect tininess before
rounding.
If you are interested you can download and run the simulator and test this out
with a docker image pulled from docker hub using the following:
# using podman instead of docker, you can use docker here too
podman pull stffrdhrn/or1k-sim-env:latest
podman run -it --rm stffrdhrn/or1k-sim-env:latest
root@9a4a52eec8ee:/tmp# or1k-elf-sim -version
Seeding random generator with value 0x4a3c2bbd
OpenRISC 1000 Architectural Simulator, version 2023-08-20
This starts up an environment which has access to the OpenRISC architecture
simulator and a GNU compiler toolchain. While still in the container can run a
quick test using the FPU as follows:
# Create a test program using OpenRISC FPU
cat > fpee.c <<EOF
#include <float.h>
#include <stdio.h>
#include <or1k-sprs.h>
#include <or1k-support.h>
static void enter_user_mode() {
int32_t sr = or1k_mfspr(OR1K_SPR_SYS_SR_ADDR);
sr &= ~OR1K_SPR_SYS_SR_SM_MASK;
or1k_mtspr(OR1K_SPR_SYS_SR_ADDR, sr);
}
static void enable_fpu_exceptions() {
unsigned long fpcsr = OR1K_SPR_SYS_FPCSR_FPEE_MASK;
or1k_mtspr(OR1K_SPR_SYS_FPCSR_ADDR, fpcsr);
}
static void fpe_handler() {
printf("Got FPU Exception, PC: 0x%lx\n", or1k_mfspr(OR1K_SPR_SYS_EPCR_BASE));
}
int main() {
float result;
or1k_exception_handler_add(0xd, fpe_handler);
#ifdef USER_MODE
/* Note, printf here also allocates some memory allowing user mode runtime to
work. */
printf("Enabling user mode\n");
enter_user_mode();
#endif
enable_fpu_exceptions();
printf("Exceptions enabled, now DIV 3.14 / 0!\n");
result = 3.14f / 0.0f;
/* Verify we see infinity. */
printf("Result: %f\n", result);
/* Verify we see DZF set. */
printf("FPCSR: %x\n", or1k_mfspr(OR1K_SPR_SYS_FPCSR_ADDR));
#ifdef USER_MODE
asm volatile("l.movhi r3, 0; l.nop 1"); /* Exit sim, now */
#endif
return 0;
}
EOF
# Compile the program
or1k-elf-gcc -g -O2 -mhard-float fpee.c -o fpee
or1k-elf-sim -f /opt/or1k/sim.cfg ./fpee
# Expected results
# Program Header: PT_LOAD, vaddr: 0x00000000, paddr: 0x0 offset: 0x00002000, filesz: 0x000065ab, memsz: 0x000065ab
# Program Header: PT_LOAD, vaddr: 0x000085ac, paddr: 0x85ac offset: 0x000085ac, filesz: 0x000000c8, memsz: 0x0000046c
# WARNING: sim_init: Debug module not enabled, cannot start remote service to GDB
# Exceptions enabled, now DIV 3.14 / 0!
# Got FPU Exception, PC: 0x2068
# Result: f
# FPCSR: 801
# Compile the program to run in USER_MODE
or1k-elf-gcc -g -O2 -mhard-float -DUSER_MODE fpee.c -o fpee
or1k-elf-sim -f /opt/or1k/sim.cfg ./fpee
# Expected results with USER_MODE
# Program Header: PT_LOAD, vaddr: 0x00000000, paddr: 0x0 offset: 0x00002000, filesz: 0x000065ab, memsz: 0x000065ab
# Program Header: PT_LOAD, vaddr: 0x000085ac, paddr: 0x85ac offset: 0x000085ac, filesz: 0x000000c8, memsz: 0x0000046c
# WARNING: sim_init: Debug module not enabled, cannot start remote service to GDB
# Enabling user mode
# Exceptions enabled, now DIV 3.14 / 0!
# Got FPU Exception, PC: 0x2068
# Result: f
# FPCSR: 801
# exit(0)
In the above we can see how to compile and run a simple FPU test program and run
it on or1ksim. The program set’s up an FPU exception handler, enables exceptions
then does a divide by zero to produce an exception. This program uses the
OpenRISC newlib (baremetal) toolchain to
compile a program that can run directly on the simulator, as oppposed to a
program running in an OS on a simulator or hardware.
Note, that normally newlib programs expect to run in supervisor mode, when
our program switches to user mode we need to take some precautions to ensure it
can run correctly. As noted in the comments, usually when allocating and exiting
the newlib runtime will do things like disabling/enabling interrupts which
will fail when running in user mode.
QEMU Updates
The QEMU update was done in my
OpenRISC user space FPCSR
qemu patch series. The series was merged for the
qemu 8.1 release.
The updates were split it into three changes:
- Allowing FPCSR access in user mode.
- Properly set the exception PC address on floating point exceptions.
- Configuring the QEMU softfloat implementation to perform tininess check
before rounding.
QEMU Patch 1
The first patch to allow FPCSR access in user mode was trivial, but required some
code structure changes making the patch look bigger than it really was.
QEMU Patch 2
The next patch to properly set the exception PC address fixed a long existing
bug where the EPCR
was not properly updated after FPU exceptions. Up until now
OpenRISC userspace did not support FPU instructions and this code path had not
been tested.
To explain why this fix is important let us look at the EPCR
and what it is used for
in a bit more detail.
In general, when an exception occurs an OpenRISC CPU will store the program counter (PC
)
of the instruction that caused the exception into the exeption program counter address
(EPCR
). Floating point exceptions are a special case in that the EPCR
is
actually set to the next instruction to be executed, this is to avoid looping.
When the linux kernel handles a floating point exception it follows the path
0xd00 > fpe_trap_handler > do_fpe_trap. This will setup a
signal to be delivered to the user process.
The Linux OS uses the EPCR
to report the exception instruction address to
userspace via a signal
which we can see being done in do_fpe_trap
which
we can see below:
asmlinkage void do_fpe_trap(struct pt_regs *regs, unsigned long address)
{
int code = FPE_FLTUNK;
unsigned long fpcsr = regs->fpcsr;
if (fpcsr & SPR_FPCSR_IVF)
code = FPE_FLTINV;
else if (fpcsr & SPR_FPCSR_OVF)
code = FPE_FLTOVF;
else if (fpcsr & SPR_FPCSR_UNF)
code = FPE_FLTUND;
else if (fpcsr & SPR_FPCSR_DZF)
code = FPE_FLTDIV;
else if (fpcsr & SPR_FPCSR_IXF)
code = FPE_FLTRES;
/* Clear all flags */
regs->fpcsr &= ~SPR_FPCSR_ALLF;
force_sig_fault(SIGFPE, code, (void __user *)regs->pc);
}
Here we see the excption becomes a SIGFPE
signal and the exception address in
regs->pc
is passed to force_sig_fault. The PC
will be used to set the
si_addr
field of the siginfo_t
structure.
Next upon return from kernel space to user space the path is do_fpe_trap
>
_fpe_trap_handler
> ret_from_exception > resume_userspace >
work_pending > do_work_pending > restore_all.
Inside of do_work_pending
with there the signal handling is done. In explain a bit
about this in the article Unwinding a Bug - How C++ Exceptions Work.
In restore_all
we see EPCR
is returned to when exception handling is
complete. A snipped of this code is show below:
#define RESTORE_ALL \
DISABLE_INTERRUPTS(r3,r4) ;\
l.lwz r3,PT_PC(r1) ;\
l.mtspr r0,r3,SPR_EPCR_BASE ;\
l.lwz r3,PT_SR(r1) ;\
l.mtspr r0,r3,SPR_ESR_BASE ;\
l.lwz r3,PT_FPCSR(r1) ;\
l.mtspr r0,r3,SPR_FPCSR ;\
l.lwz r2,PT_GPR2(r1) ;\
l.lwz r3,PT_GPR3(r1) ;\
l.lwz r4,PT_GPR4(r1) ;\
l.lwz r5,PT_GPR5(r1) ;\
l.lwz r6,PT_GPR6(r1) ;\
l.lwz r7,PT_GPR7(r1) ;\
l.lwz r8,PT_GPR8(r1) ;\
l.lwz r9,PT_GPR9(r1) ;\
l.lwz r10,PT_GPR10(r1) ;\
l.lwz r11,PT_GPR11(r1) ;\
l.lwz r12,PT_GPR12(r1) ;\
l.lwz r13,PT_GPR13(r1) ;\
l.lwz r14,PT_GPR14(r1) ;\
l.lwz r15,PT_GPR15(r1) ;\
l.lwz r16,PT_GPR16(r1) ;\
l.lwz r17,PT_GPR17(r1) ;\
l.lwz r18,PT_GPR18(r1) ;\
l.lwz r19,PT_GPR19(r1) ;\
l.lwz r20,PT_GPR20(r1) ;\
l.lwz r21,PT_GPR21(r1) ;\
l.lwz r22,PT_GPR22(r1) ;\
l.lwz r23,PT_GPR23(r1) ;\
l.lwz r24,PT_GPR24(r1) ;\
l.lwz r25,PT_GPR25(r1) ;\
l.lwz r26,PT_GPR26(r1) ;\
l.lwz r27,PT_GPR27(r1) ;\
l.lwz r28,PT_GPR28(r1) ;\
l.lwz r29,PT_GPR29(r1) ;\
l.lwz r30,PT_GPR30(r1) ;\
l.lwz r31,PT_GPR31(r1) ;\
l.lwz r1,PT_SP(r1) ;\
l.rfe
Here we can see how l.mtspr r0,r3,SPR_EPCR_BASE
restores the EPCR
to the pc
address stored in pt_regs
when we entered the exception handler. All
other register are restored and finally the l.rfe
instruction is issued to
return from the exception which affectively jumps to EPCR
.
The reason QEMU was not setting the correct exception address is due to the way
qemu is implemented which optimizes performance. QEMU executes target code
basic blocks that are translated to host native instructions, during runtime
all PC
addresses are those of the host, for example x86-64 64-bit
addresses. When an exception occurs, updating the target PC
address from the host PC
need to be explicityly requested.
QEMU Patch 3
The next patch to implement tininess before rouding was also trivial but
brought up a conversation about default NaN payloads.
QEMU Patch 4
Wait, there is more. During writing this article I realized that if QEMU
was setting the ECPR
to the FPU instruction causing the exception then
we would end up in an endless loop.
Luckily the arcitecture anticipated this calling for FPU exceptions to set the next
instruction to be executed to EPCR
. QEMU was missing this logic.
The patch target/openrisc: Set EPCR to next PC on FPE exceptions
fixes this up.
RTL Updates
Updating the actual verilog RTL CPU implementations also needed to be done.
Updates have been made to both the mor1kx
and the
or1k_marocchino
implementations.
mor1kx Updates
Updates to the mor1kx to support user mode reads and write to the FPCSR
were done in the patch:
Make FPCSR is R/W accessible for both user- and supervisor- modes.
The full patch is:
@@ -618,7 +618,7 @@ module mor1kx_ctrl_cappuccino
spr_fpcsr[`OR1K_FPCSR_FPEE] <= 1'b0;
end
else if ((spr_we & spr_access[`OR1K_SPR_SYS_BASE] &
- (spr_sr[`OR1K_SPR_SR_SM] & padv_ctrl | du_access)) &&
+ (padv_ctrl | du_access)) &&
`SPR_OFFSET(spr_addr)==`SPR_OFFSET(`OR1K_SPR_FPCSR_ADDR)) begin
spr_fpcsr <= spr_write_dat[`OR1K_FPCSR_WIDTH-1:0]; // update all fields
`ifdef OR1K_FPCSR_MASK_FLAGS
The change to verilog shows that before when writng (spr_we
) to the FPCSR (OR1K_SPR_FPCSR_ADDR
) register
we used to check that the supervisor bit (OR1K_SPR_SR_SM
) bit of the sr spr (spr_sr
) is set. That check
enforced supervisor mode only write access, removing this allows user space to write to the regsiter.
Updating mor1kx to support tininess checking before rounding was done in the
change Refactoring and implementation tininess detection before
rounding.
I will not go into the details of these patches as I don’t understand them so
much.
Marocchino Updates
Updates to the or1k_marocchino to support user mode reads and write to the FPCSR
were done in the patch:
Make FPCSR is R/W accessible for both user- and supervisor- modes.
The full patch is:
@@ -714,7 +714,7 @@ module or1k_marocchino_ctrl
assign except_fpu_enable_o = spr_fpcsr[`OR1K_FPCSR_FPEE];
wire spr_fpcsr_we = (`SPR_OFFSET(({1'b0, spr_sys_group_wadr_r})) == `SPR_OFFSET(`OR1K_SPR_FPCSR_ADDR)) &
- spr_sys_group_we & spr_sr[`OR1K_SPR_SR_SM];
+ spr_sys_group_we; // FPCSR is R/W for both user- and supervisor- modes
`ifdef OR1K_FPCSR_MASK_FLAGS
reg [`OR1K_FPCSR_ALLF_SIZE-1:0] ctrl_fpu_mask_flags_r;
Updating the marocchino to support dttectig tininess before rounding was done in the
patch:
Refactoring FPU Implementation for tininess detection BEFORE ROUNDING.
I will not go into details of the patch as I didn’t write them. In
general it is a medium size refactoring of the floating point unit.
Summary
We discussed updates to the architecture simulators and verilog CPU implementations
to allow supporting user mode floating point programs. These updates will now allow us to
port Linux and glibc to the OpenRISC floating point unit.
Further Reading
25 Apr 2023
Last year (2022) the big milestone for OpenRISC was getting the glibc port upstream.
Though there is libc support for
OpenRISC already with musl and ucLibc
the glibc port provides a extensive testsuite which has proved useful in shaking out toolchain
and OS bugs.
The upstreamed OpenRISC glibc support is missing support for leveraging the
OpenRISC floating-point unit (FPU).
Adding OpenRISC glibc FPU support requires a cross cutting effort across the
architecture’s fullstack from:
- Architecture Specification
- Simulators and CPU implementations
- Linux Kernel support
- GCC Instructions and Soft FPU
- Binutils/GDB Debugging Support
- glibc support
In this blog entry I will cover how the OpenRISC architecture specification
was updated to support user space floating point applications. But first, what
is FPU porting?
What is FPU Porting?
The FPU in modern CPU’s allow the processor to perform IEEE 754
floating point math like addition, subtraction, multiplication. When used in a
user application the FPU’s function becomes more of a math accelerator, speeding
up math operations including
trigonometric and
complex functions such as sin
,
sinf
and cexpf
. Not all FPU’s provide the same
set of FPU operations nor do they have to. When enabled, the compiler will
insert floating point instructions where they can be used.
OpenRISC FPU support was added to the GCC compiler a while back.
We can see how this works with a simple example using the bare-metal newlib toolchain.
C code example addf.c
:
float addf(float a, float b) {
return a + b;
}
To compile this C function we can do:
$ or1k-elf-gcc -O2 addf.c -c -o addf-sf.o
$ or1k-elf-gcc -O2 -mhard-float addf.c -c -o addf-hf.o
Assembly output of addf-sf.o
contains the default software floating point
implementation as we can see below. We can see below that a call to __addsf3
was
added to perform our floating point operation. The function __addsf3
is provided
by libgcc
as a software implementation of the single precision
floating point (sf
) add operation.
$ or1k-elf-objdump -dr addf-sf.o
Disassembly of section .text:
00000000 <addf>:
0: 9c 21 ff fc l.addi r1,r1,-4
4: d4 01 48 00 l.sw 0(r1),r9
8: 04 00 00 00 l.jal 8 <addf+0x8>
8: R_OR1K_INSN_REL_26 __addsf3
c: 15 00 00 00 l.nop 0x0
10: 85 21 00 00 l.lwz r9,0(r1)
14: 44 00 48 00 l.jr r9
18: 9c 21 00 04 l.addi r1,r1,4
The disassembly of the addf-hf.o
below shows that the FPU instruction
(hardware) lf.add.s
is used to perform addition, this is because the snippet
was compiled using the -mhard-float
argument. One could imagine if this is
supported it would be more efficient compared to the software implementation.
$ or1k-elf-objdump -dr addf-hf.o
Disassembly of section .text:
00000000 <addf>:
0: c9 63 20 00 lf.add.s r11,r3,r4
4: 44 00 48 00 l.jr r9
8: 15 00 00 00 l.nop 0x0
So if the OpenRISC toolchain already has support for FPU instructions what else
needs to be done? When we add FPU support to glibc we are adding FPU support
to the OpenRISC POSIX runtime and create a toolchain that can compile and link
binaries to run on this runtime.
The Runtime
Below we can see examples of two application runtimes, one Application A runs
with software floating point, the other Application B run’s with full hardware
floating point.
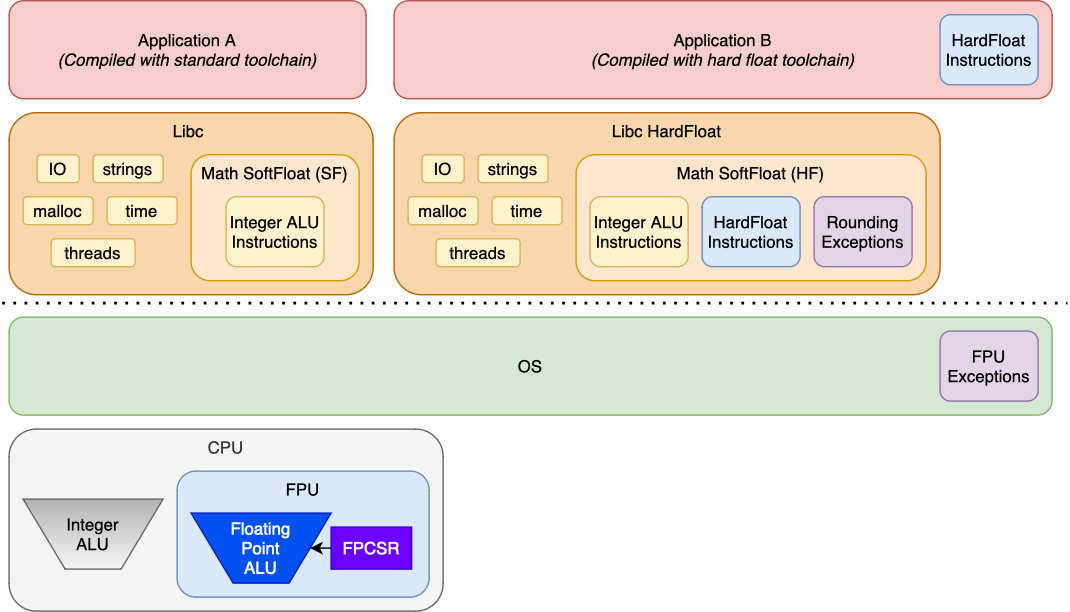
Both Application A and Application B can run on the same system, but
Application B requires a libc and kernel that support the floating point
runtime. As we can see:
- In Application B it leverages floating point instructions as noted in the
blue box. That should be implemented in the
CPU, and are produced by the GCC compiler.
- The math routines in the C Library used by Application B are accelarated by
the FPU as per the blue box. The math routines can also set up rounding of the FPU hardware to
be in line with rounding of the software routines. The math routines can
also detect exceptions by checking the FPU state. The rounding and exception
handling in the purple boxes is what is
implemented the GLIBC.
- The kernel must be able to save and restore the FPU state when switching
between processes. The OS also has support for signalling the process if
enabled. This is indicated in the purple
box.
Another aspect is that supporting hardware floating point in the OS means that
multiple user land programs can transparently use the FPU. To do all of this we
need to update the kernel and the C runtime libraries to:
- Make the kernel save and restore process FPU state during context switches
- Make the kernel handle FPU exceptions and deliver signals to user land
- Teach GLIBC how to setup FPU rounding mode
- Teach GLIBC how to translate FPU exceptions
- Tell GCC and GLIBC soft float about our FPU quirks
In order to compile applications like Application B a separate compiler
toolchain is needed. For highly configurable embredded system CPU’s like ARM, RISC-V there
are multiple toolchains available for building software for the different CPU
configurations. Usually there will be one toolchain for soft float and one for hard float support, see the below example
from the arm toolchain download page.
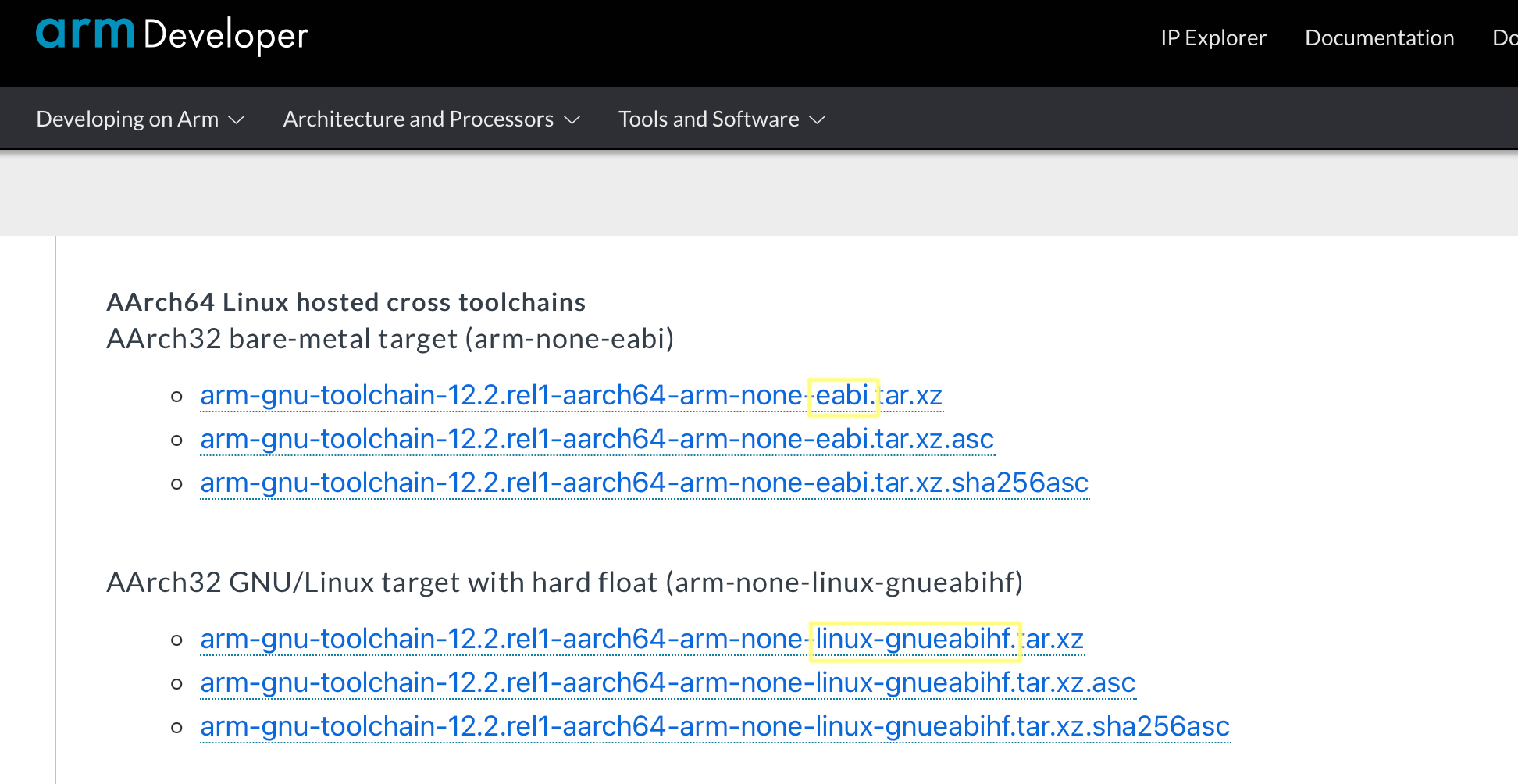
Fixing Architecture Issues
As we started to work on the floating point support we found two issues:
- The OpenRISC floating point control and status register (FPCSR) is accessible only in
supervisor mode.
- We have not defined how the FPU should perform tininess detection.
FPCSR Access
The GLIBC OpenRISC FPU port, or any port for that matter, starts
by looking at what other architectures have done. For GLIBC FPU support we can
look at what MIPS, ARM, RISC-V etc. have implemented. Most ports have a file
called sysdeps/{arch}/fpu_control.h
, I noticed one thing right away as I went
through this, we can look at ARM or MIPS for example:
sysdeps/mips/fpu_control.h:
Excerpt from the MIPS port showing the definition of _FPU_GETCW and _FPU_SETCW
#else
# define _FPU_GETCW(cw) __asm__ volatile ("cfc1 %0,$31" : "=r" (cw))
# define _FPU_SETCW(cw) __asm__ volatile ("ctc1 %0,$31" : : "r" (cw))
#endif
sysdeps/arm/fpu_control.h:
Excerpt from the ARM port showing the definition of _FPU_GETCW and _FPU_SETCW
# define _FPU_GETCW(cw) \
__asm__ __volatile__ ("vmrs %0, fpscr" : "=r" (cw))
# define _FPU_SETCW(cw) \
__asm__ __volatile__ ("vmsr fpscr, %0" : : "r" (cw))
#endif
What we see here is a macro that defines how to read or write the floating point
control word for each architecture. The macros are implemented using a single
assembly instruction.
In OpenRISC we have similar instructions for reading and writing the floating
point control register (FPCSR), writing for example is: l.mtspr r0,%0,20
. However,
on OpenRISC the FPCSR is read-only when running in user-space, this is a
problem.
If we remember from our operating system studies, user applications run in
user-mode as
apposed to the privileged kernel-mode.
The user floating point environment
is defined by POSIX in the ISO C Standard. The C library provides functions to
set rounding modes and clear exceptions using for example
fesetround
for setting FPU rounding modes and
feholdexcept for clearing exceptions.
If userspace applications need to be able to control the floating point unit
the having architectures support for this is integral.
Originally OpenRISC architecture specification
specified the floating point control and status registers (FPCSR) as being
read only when executing in user mode, again this is a problem and needs to
be addressed.
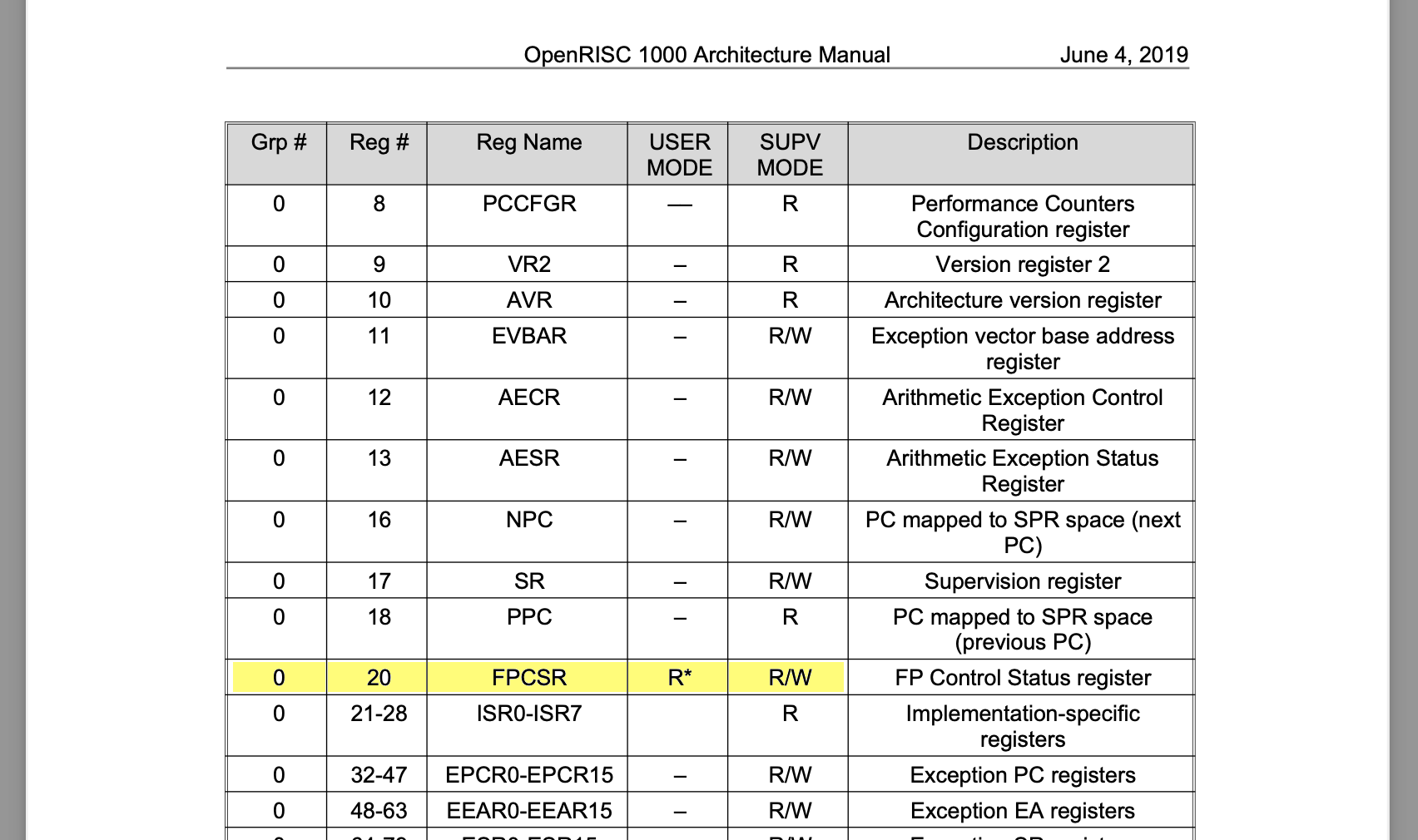
Other architectures define the floating point control register as being writable in user-mode.
For example, ARM has the
FPCR and FPSR,
and RISC-V has the
FCSR
all of which are writable in user-mode.
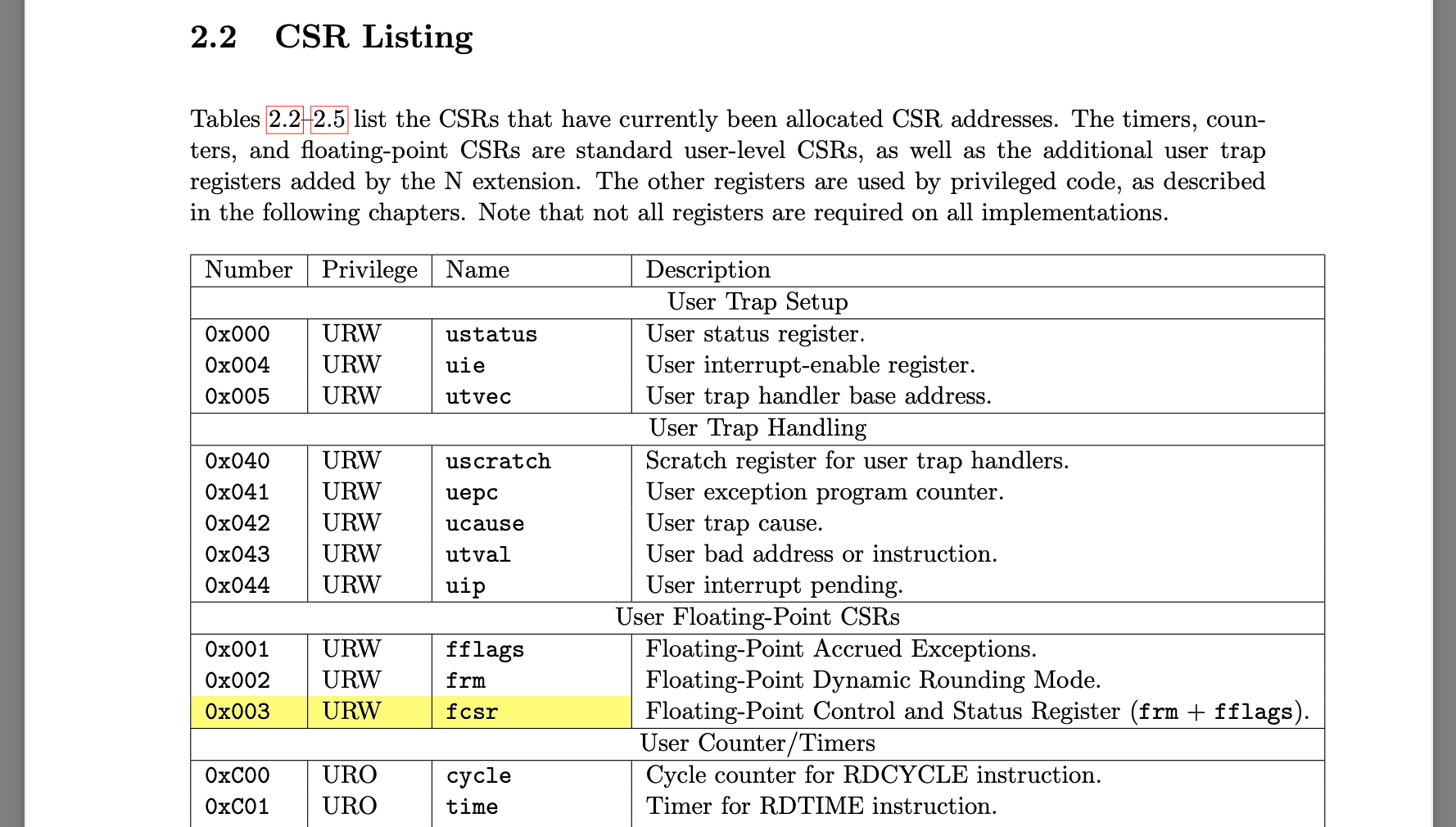
Tininess Detection
I am skipping ahead a bit here, once the OpenRISC GLIBC port was working we noticed
many problematic math test failures. This turned out to be inconsistencies
between the tininess detection [pdf]
settings in the toolchain. Tininess detection must be selected by an FPU
implementation as being done before or after rounding.
In the toolchain this is configured by:
- GLIBC
TININESS_AFTER_ROUNDING
- macro used by test suite to control
expectations
- GLIBC
_FP_TININESS_AFTER_ROUNDING
- macro used to control softfloat
implementation in GLIBC.
- GCC libgcc
_FP_TININESS_AFTER_ROUNDING
- macro used to control softfloat
implementation in GCC libgcc.
Updating the Spec
Writing to FPCSR from user-mode could be worked around in OpenRISC by
introducing a syscall, but we decided to just change the architecture
specification for this. Updating the spec keeps it similar to all other
architectures out there.
In OpenRISC we have defined tininess detection to be done before rounding as
this matches what existing FPU implementation have done.
As of architecture specification revision
1.4 the FPCSR is defined as being writable
in user-mode and we have documented tininess detection to be before rounding.
Summary
We’ve gone through an overview of how the FPU accelarates math in
an application runtime. We then looked how the OpenRISC architecture specification needed
to be updated to support the floating point POSIX runtime.
In the next entry we shall look into patches to get QEMU and and CPU
implementations updated to support the new spec changes.
13 Dec 2020
I have been working on porting GLIBC to the OpenRISC architecture. This has
taken longer than I expected as with GLIBC upstreaming we must get every
test to pass. This was different compared to GDB and GCC which were a
bit more lenient.
My first upstreaming attempt
was completely tested on the QEMU simulator. I have
since added an FPGA LiteX SoC
to my test platform options. LiteX runs Linux on the OpenRISC mor1kx softcore
and tests are loaded over an SSH session. The SoC eliminates an issue I was
seeing on the simulator where under heavy load it appears the MMU starves the kernel
from getting any work done.
To get to where I am now this required:
Adding GDB Linux debugging support is great because it allows debugging of
multithreaded processes and signal handling; which we are going to need.
A Bug
Our story starts when I was trying to fix a failing GLIBC NPTL
test case. The test case involves C++ exceptions and POSIX threads.
The issue is that the catch
block of a try/catch
block is not
being called. Where do we even start?
My plan for approaching test case failures is:
- Understand what the test case is trying to test and where its failing
- Create a hypothesis about where the problem is
- Understand how the failing API’s works internally
- Debug until we find the issue
- If we get stuck go back to
2.
Let’s have a try.
Understanding the Test case
The GLIBC test case is nptl/tst-cancel24.cc.
The test starts in the do_test
function and it will create a child thread with pthread_create
.
The child thread executes function tf
which waits on a semaphore until the parent thread cancels it. It
is expected that the child thread, when cancelled , will call it’s catch block.
The failure is that the catch
block is not getting run as evidenced by the except_caught
variable
not being set to true
.
Below is an excerpt from the test showing the tf
function.
static void *
tf (void *arg) {
sem_t *s = static_cast<sem_t *> (arg);
try {
monitor m;
pthread_barrier_wait (&b);
while (1)
sem_wait (s);
} catch (...) {
except_caught = true;
throw;
}
return NULL;
}
So the catch
block is not being run. Simple, but where do we start to
debug that? Let’s move onto the next step.
Creating a Hypothesis
This one is a bit tricky as it seems C++ try/catch
blocks are broken. Here, I am
working on GLIBC testing, what does that have to do with C++?
To get a better idea of where the problem is I tried to modify the test to test
some simple ideas. First, maybe there is a problem with catching exceptions
throws from thread child functions.
static void do_throw() { throw 99; }
static void * tf () {
try {
monitor m;
while (1) do_throw();
} catch (...) {
except_caught = true;
}
return NULL;
}
No, this works correctly. So try/catch
is working.
Hypothesis: There is a problem handling exceptions while in a syscall.
There may be something broken with OpenRISC related to how we setup stack
frames for syscalls that makes the unwinder fail.
How does that work? Let’s move onto the next step.
Understanding the Internals
To find this bug we need to understand how C++ exceptions work. Also, we need to know
what happens when a thread is cancelled in a multithreaded
(pthread) glibc environment.
There are a few contributors pthread cancellation and C++ exceptions which are:
- DWARF - provided by our program and libraries in the
.eh_frame
ELF
section
- GLIBC - provides the pthread runtime and cleanup callbacks to the GCC unwinder code
- GCC - provides libraries for dealing with exceptions
libgcc_s.so
- handles unwinding by reading program DWARF metadata and doing the frame decoding
libstdc++.so.6
- provides the C++ personality routine which
identifies and prepares catch
blocks for execution
DWARF
ELF binaries provide debugging information in a data format called
DWARF. The name was chosen to maintain a
fantasy theme. Lately the Linux community has a new debug format called
ORC.
Though DWARF is a debugging format and usually stored in .debug_frame
,
.debug_info
, etc sections, a stripped down version it is used for exception
handling.
Each ELF binary that supports unwinding contains the .eh_frame
section to
provide unwinding information. This can be seen with the readelf
program.
$ readelf -S sysroot/lib/libc.so.6
There are 70 section headers, starting at offset 0xaa00b8:
Section Headers:
[Nr] Name Type Addr Off Size ES Flg Lk Inf Al
[ 0] NULL 00000000 000000 000000 00 0 0 0
[ 1] .note.ABI-tag NOTE 00000174 000174 000020 00 A 0 0 4
[ 2] .gnu.hash GNU_HASH 00000194 000194 00380c 04 A 3 0 4
[ 3] .dynsym DYNSYM 000039a0 0039a0 008280 10 A 4 15 4
[ 4] .dynstr STRTAB 0000bc20 00bc20 0054d4 00 A 0 0 1
[ 5] .gnu.version VERSYM 000110f4 0110f4 001050 02 A 3 0 2
[ 6] .gnu.version_d VERDEF 00012144 012144 000080 00 A 4 4 4
[ 7] .gnu.version_r VERNEED 000121c4 0121c4 000030 00 A 4 1 4
[ 8] .rela.dyn RELA 000121f4 0121f4 00378c 0c A 3 0 4
[ 9] .rela.plt RELA 00015980 015980 000090 0c AI 3 28 4
[10] .plt PROGBITS 00015a10 015a10 0000d0 04 AX 0 0 4
[11] .text PROGBITS 00015ae0 015ae0 155b78 00 AX 0 0 4
[12] __libc_freeres_fn PROGBITS 0016b658 16b658 001980 00 AX 0 0 4
[13] .rodata PROGBITS 0016cfd8 16cfd8 0192b4 00 A 0 0 4
[14] .interp PROGBITS 0018628c 18628c 000018 00 A 0 0 1
[15] .eh_frame_hdr PROGBITS 001862a4 1862a4 001a44 00 A 0 0 4
[16] .eh_frame PROGBITS 00187ce8 187ce8 007cf4 00 A 0 0 4
[17] .gcc_except_table PROGBITS 0018f9dc 18f9dc 000341 00 A 0 0 1
...
We can decode the metadata using readelf
as well using the
--debug-dump=frames-interp
and --debug-dump=frames
arguments.
The frames
dump provides a raw output of the DWARF metadata for each frame.
This is not usually as useful as frames-interp
, but it shows how the DWARF
format is actually a bytecode. The DWARF interpreter needs to execute these
operations to understand how to derive the values of registers based current PC.
There is an interesting talk in Exploiting the hard-working
DWARF.pdf.
An example of the frames
dump:
$ readelf --debug-dump=frames sysroot/lib/libc.so.6
...
00016788 0000000c ffffffff CIE
Version: 1
Augmentation: ""
Code alignment factor: 4
Data alignment factor: -4
Return address column: 9
DW_CFA_def_cfa_register: r1
DW_CFA_nop
00016798 00000028 00016788 FDE cie=00016788 pc=0016b584..0016b658
DW_CFA_advance_loc: 4 to 0016b588
DW_CFA_def_cfa_offset: 4
DW_CFA_advance_loc: 8 to 0016b590
DW_CFA_offset: r9 at cfa-4
DW_CFA_advance_loc: 68 to 0016b5d4
DW_CFA_remember_state
DW_CFA_def_cfa_offset: 0
DW_CFA_restore: r9
DW_CFA_restore_state
DW_CFA_advance_loc: 56 to 0016b60c
DW_CFA_remember_state
DW_CFA_def_cfa_offset: 0
DW_CFA_restore: r9
DW_CFA_restore_state
DW_CFA_advance_loc: 36 to 0016b630
DW_CFA_remember_state
DW_CFA_def_cfa_offset: 0
DW_CFA_restore: r9
DW_CFA_restore_state
DW_CFA_advance_loc: 40 to 0016b658
DW_CFA_def_cfa_offset: 0
DW_CFA_restore: r9
The frames-interp
argument is a bit more clear as it shows the interpreted output
of the bytecode. Below we see two types of entries:
CIE
- Common Information Entry
FDE
- Frame Description Entry
The CIE
provides starting point information for each child FDE
entry. Some
things to point out: we see ra=9
indicates the return address is stored in
register r9
, we see CFA r1+0
indicates the canonical frame pointer is stored in
register r1
and we see the stack frame size is 4
bytes.
An example of the frames-interp
dump:
$ readelf --debug-dump=frames-interp sysroot/lib/libc.so.6
...
00016788 0000000c ffffffff CIE "" cf=4 df=-4 ra=9
LOC CFA
00000000 r1+0
00016798 00000028 00016788 FDE cie=00016788 pc=0016b584..0016b658
LOC CFA ra
0016b584 r1+0 u
0016b588 r1+4 u
0016b590 r1+4 c-4
0016b5d4 r1+4 c-4
0016b60c r1+4 c-4
0016b630 r1+4 c-4
0016b658 r1+0 u
GLIBC
GLIBC provides pthreads
which when used with C++ needs to support exception
handling. The main place exceptions are used with pthreads
is when cancelling
threads. When using pthread_cancel
a cancel signal is sent to the target thread using tgkill
which causes an exception.
This is implemented with the below APIs.
- sigcancel_handler -
Setup during the pthread runtime initialization, it handles cancellation,
which calls
__do_cancel
, which calls __pthread_unwind
.
- __pthread_unwind -
Is called with
pd->cancel_jmp_buf
. It calls glibc’s __Unwind_ForcedUnwind
.
- _Unwind_ForcedUnwind -
Loads GCC’s
libgcc_s.so
version of _Unwind_ForcedUnwind
and calls it with parameters:
exc
- the exception context
unwind_stop
- the stop callback to GLIBC, called for each frame of the unwind, with
the stop argument ibuf
ibuf
- the jmp_buf
, created by setjmp
(self->cancel_jmp_buf
) in start_thread
- unwind_stop -
Checks the current state of unwind and call the
cancel_jmp_buf
if
we are at the end of stack. When the cancel_jmp_buf
is called the thread
exits.
Let’s look at pd->cancel_jmp_buf
in more details. The cancel_jmp_buf
is
setup during pthread_create
after clone in start_thread.
It uses the setjmp and
longjump non local goto mechanism.
Let’s look at some diagrams.
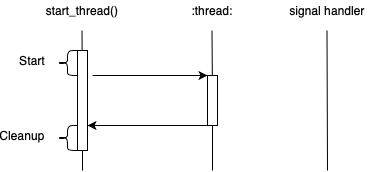
The above diagram shows a pthread that exits normally. During the Start phase
of the thread setjmp
will create the cancel_jmp_buf
. After the thread
routine exits it returns to the start_thread
routine to do cleanup.
The cancel_jmp_buf
is not used.
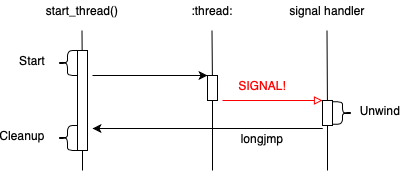
The above diagram shows a pthread that is cancelled. When the
thread is created setjmp
will create the cancel_jmp_buf
. In this case
while the thread routine is running it is cancelled, the unwinder runs
and at the end it calls unwind_stop
which calls longjmp
. After the
longjmp
the thread is returned to start_thread
to do cleanup.
A highly redacted version of our start_thread
and unwind_stop
functions is
shown below.
start_thread()
{
struct pthread *pd = START_THREAD_SELF;
...
struct pthread_unwind_buf unwind_buf;
int not_first_call;
not_first_call = setjmp ((struct __jmp_buf_tag *) unwind_buf.cancel_jmp_buf);
...
if (__glibc_likely (! not_first_call))
{
/* Store the new cleanup handler info. */
THREAD_SETMEM (pd, cleanup_jmp_buf, &unwind_buf);
...
/* Run the user provided thread routine */
ret = pd->start_routine (pd->arg);
THREAD_SETMEM (pd, result, ret);
}
... free resources ...
__exit_thread ();
}
unwind_stop (_Unwind_Action actions,
struct _Unwind_Context *context, void *stop_parameter)
{
struct pthread_unwind_buf *buf = stop_parameter;
struct pthread *self = THREAD_SELF;
int do_longjump = 0;
...
if ((actions & _UA_END_OF_STACK)
|| ... )
do_longjump = 1;
...
/* If we are at the end, go back start_thread for cleanup */
if (do_longjump)
__libc_unwind_longjmp ((struct __jmp_buf_tag *) buf->cancel_jmp_buf, 1);
return _URC_NO_REASON;
}
GCC
GCC provides the exception handling and unwinding capabilities
to the C++ runtime. They are provided in the libgcc_s.so
and libstdc++.so.6
libraries.
The libgcc_s.so
library implements the IA-64 Itanium Exception Handling ABI.
It’s interesting that the now defunct Itanium
architecture introduced this ABI which is now the standard for all processor exception
handling. There are two main entry points for the unwinder are:
_Unwind_ForcedUnwind
- for forced unwinding
_Unwind_RaiseException
- for raising normal exceptions
There are also two data structures to be aware of:
- _Unwind_Context - register and unwind state for a frame, below referenced as CONTEXT
- _Unwind_FrameState - register and unwind state from DWARF, below referenced as FS
The _Unwind_Context
important parts:
struct _Unwind_Context {
_Unwind_Context_Reg_Val reg[__LIBGCC_DWARF_FRAME_REGISTERS__+1];
void *cfa;
void *ra;
struct dwarf_eh_bases bases;
_Unwind_Word flags;
};
The _Unwind_FrameState
important parts:
typedef struct {
struct frame_state_reg_info { ... } regs;
void *pc;
/* The information we care about from the CIE/FDE. */
_Unwind_Personality_Fn personality;
_Unwind_Sword data_align;
_Unwind_Word code_align;
_Unwind_Word retaddr_column;
unsigned char fde_encoding;
unsigned char signal_frame;
void *eh_ptr;
} _Unwind_FrameState;
These two data structures are very similar. The _Unwind_FrameState
is for internal
use and closely ties to the DWARF definitions of the frame. The _Unwind_Context
struct is more generic and is used as an opaque structure in the public unwind api.
Forced Unwinds
Exceptions that are raised for thread cancellation use a single phase forced unwind.
Code execution will not resume, but catch blocks will be run. This is why
cancel exceptions must be rethrown.
Forced unwinds use the unwind_stop
handler which GLIBC provides as explained in
the GLIBC section above.
- _Unwind_ForcedUnwind - calls:
_Unwind_ForcedUnwind_Phase2
- loops forever doing:
- uw_frame_state_for - populate FS for the frame one frame above CONTEXT, searching DWARF using CONTEXT->ra
stop
- callback to GLIBC to stop the unwind if needed
FS.personality
- the C++ personality routine, see below, called with _UA_FORCE_UNWIND | _UA_CLEANUP_PHASE
- uw_advance_context - advance CONTEXT by populating it from FS
Normal Exceptions
For exceptions raised programmatically unwinding is very similar to the forced unwind, but
there is no stop
function and exception unwinding is 2 phase.
- _Unwind_RaiseException - calls:
uw_init_context
- load details of the current frame from cpu/stack into CONTEXT
- Do phase 1 loop:
uw_frame_state_for
- populate FS for the frame one frame above CONTEXT, searching DWARF using CONTEXT->ra
FS.personality
- the C++ personality routine, see below, called with _UA_SEARCH_PHASE
- uw_update_context - advance CONTEXT by populating it from FS (same as
uw_advance_context
)
- _Unwind_RaiseException_Phase2 - do the frame iterations
uw_install_context
- exit unwinder jumping to selected frame
_Unwind_RaiseException_Phase2
- do phase 2, loops forever doing:
uw_frame_state_for
- populate FS for the frame one frame above CONTEXT, searching DWARF using CONTEXT->ra
FS.personality
- the C++ personality routine, called with _UA_CLEANUP_PHASE
uw_update_context
- advance CONTEXT by populating it from FS
The libstdc++.so.6
library provides the C++ standard library
which includes the C++ personality routine __gxx_personality_v0.
The personality routine is the interface between the unwind routines and the c++
(or other language) runtime, which handles the exception handling logic for that
language.
As we saw above the personality routine is executed for each stack frame. The
function checks if there is a catch
block that matches the exception being
thrown. If there is a match, it will update the context to prepare it to jump
into the catch routine and return _URC_INSTALL_CONTEXT
. If there is no catch
block matching it returns _URC_CONTINUE_UNWIND
.
In the case of _URC_INSTALL_CONTEXT
then the _Unwind_ForcedUnwind_Phase2
loop breaks and calls uw_install_context
.
Unwinding through a Signal Frame
When the GCC unwinder is looping through frames the uw_frame_state_for
function will search DWARF information. The DWARF lookup will fail for signal
frames and a fallback mechanism is provided for each architecture to handle
this. For OpenRISC Linux this is handled by
or1k_fallback_frame_state.
To understand how this works let’s look into the Linux kernel a bit.
A process must be context switched to kernel by either a system call, timer or other
interrupt in order to receive a signal.
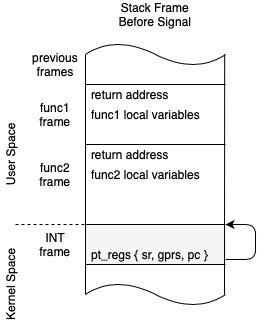
The diagram above shows what a process stack looks like after the kernel takes over.
An interrupt frame is push to the top of the stack and the pt_regs
structure
is filled out containing the processor state before the interrupt.
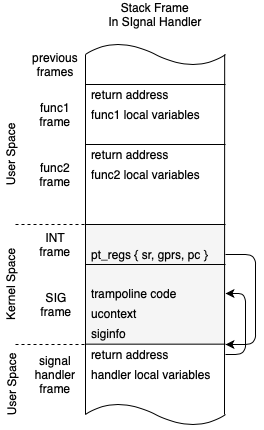
This second diagram shows what happens when a signal handler is invoked. A new
special signal frame is pushed onto the stack and when the process is resumed
it resumes in the signal handler. In OpenRISC the signal frame is setup by the setup_rt_frame
function which is called inside of do_signal
which calls handle_signal
which calls setup_rt_frame
.
After the signal handler routine runs we return to a special bit of code called
the Trampoline. The trampoline code lives on the stack and runs
sigretrun.
Now back to or1k_fallback_frame_state
.
The or1k_fallback_frame_state
function checks if the current frame is a
signal frame by confirming the return address points to a Trampoline. If
it is a trampoline it looks into the kernel saved ucontext
and pt_regs
find
the previous user frame. Unwinding, can then continue as normal.
Debugging the Issue
Now with a good background in how unwinding works we can start to debug our test
case. We can recall our hypothesis:
Hypothesis: There is a problem handling exceptions while in a syscall.
There may be something broken with OpenRISC related to how we setup stack
frames for syscalls that makes the unwinder fail.
With GDB we can start to debug exception handling, we can trace right to the
start of the exception handling logic by setting our breakpoint at
_Unwind_ForcedUnwind
.
This is the stack trace we see:
#0 _Unwind_ForcedUnwind_Phase2 (exc=0x30caf658, context=0x30caeb6c, frames_p=0x30caea90) at ../../../libgcc/unwind.inc:192
#1 0x30303858 in _Unwind_ForcedUnwind (exc=0x30caf658, stop=0x30321dcc <unwind_stop>, stop_argument=0x30caeea4) at ../../../libgcc/unwind.inc:217
#2 0x30321fc0 in __GI___pthread_unwind (buf=<optimized out>) at unwind.c:121
#3 0x30312388 in __do_cancel () at pthreadP.h:313
#4 sigcancel_handler (sig=32, si=0x30caec98, ctx=<optimized out>) at nptl-init.c:162
#5 sigcancel_handler (sig=<optimized out>, si=0x30caec98, ctx=<optimized out>) at nptl-init.c:127
#6 <signal handler called>
#7 0x303266d0 in __futex_abstimed_wait_cancelable64 (futex_word=0x7ffffd78, expected=1, clockid=<optimized out>, abstime=0x0, private=<optimized out>)
at ../sysdeps/nptl/futex-internal.c:66
#8 0x303210f8 in __new_sem_wait_slow64 (sem=0x7ffffd78, abstime=0x0, clockid=0) at sem_waitcommon.c:285
#9 0x00002884 in tf (arg=0x7ffffd78) at throw-pthread-sem.cc:35
#10 0x30314548 in start_thread (arg=<optimized out>) at pthread_create.c:463
#11 0x3043638c in __or1k_clone () from /lib/libc.so.6
Backtrace stopped: frame did not save the PC
(gdb)
In the GDB backtrack we can see it unwinds through, the signal frame, sem_wait
all the way to our thread routine tf
. It appears everything, is working fine.
But we need to remember the backtrace we see above is from GDB’s unwinder not
GCC, also it uses the .debug_info
DWARF data, not .eh_frame
.
To really ensure the GCC unwinder is working as expected we need to debug it
walking the stack. Debugging when we unwind a signal frame can be done by
placing a breakpoint on or1k_fallback_frame_state
.
Debugging this code as well shows it works correctly.
#0 or1k_fallback_frame_state (context=<optimized out>, context=<optimized out>, fs=<optimized out>) at ../../../libgcc/unwind-dw2.c:1271
#1 uw_frame_state_for (context=0x30caeb6c, fs=0x30cae914) at ../../../libgcc/unwind-dw2.c:1271
#2 0x30303200 in _Unwind_ForcedUnwind_Phase2 (exc=0x30caf658, context=0x30caeb6c, frames_p=0x30caea90) at ../../../libgcc/unwind.inc:162
#3 0x30303858 in _Unwind_ForcedUnwind (exc=0x30caf658, stop=0x30321dcc <unwind_stop>, stop_argument=0x30caeea4) at ../../../libgcc/unwind.inc:217
#4 0x30321fc0 in __GI___pthread_unwind (buf=<optimized out>) at unwind.c:121
#5 0x30312388 in __do_cancel () at pthreadP.h:313
#6 sigcancel_handler (sig=32, si=0x30caec98, ctx=<optimized out>) at nptl-init.c:162
#7 sigcancel_handler (sig=<optimized out>, si=0x30caec98, ctx=<optimized out>) at nptl-init.c:127
#8 <signal handler called>
#9 0x303266d0 in __futex_abstimed_wait_cancelable64 (futex_word=0x7ffffd78, expected=1, clockid=<optimized out>, abstime=0x0, private=<optimized out>) at ../sysdeps/nptl/futex-internal.c:66
#10 0x303210f8 in __new_sem_wait_slow64 (sem=0x7ffffd78, abstime=0x0, clockid=0) at sem_waitcommon.c:285
#11 0x00002884 in tf (arg=0x7ffffd78) at throw-pthread-sem.cc:35
Debugging when the unwinding stops can be done by setting a breakpoint
on the unwind_stop
function.
When debugging I was able to see that the unwinder failed when looking for
the __futex_abstimed_wait_cancelable64
frame. So, this is not an issue
with unwinding signal frames.
A second Hypothosis
Debugging showed that the uwinder is working correctly, and it can properly
unwind through our signal frames. However, the unwinder is bailing out early
before it gets to the tf
frame which has the catch block we need to execute.
Hypothesis 2: There is something wrong finding DWARF info for __futex_abstimed_wait_cancelable64
.
Looking at libpthread.so
with readelf
this function was missing completely from the .eh_frame
metadata. Now we found something.
What creates the .eh_frame
anyway? GCC or Binutils (Assembler). If we run GCC
with the -S
argument we can see GCC will output inline .cfi
directives.
These .cfi
annotations are what gets compiled to the to .eh_frame
. GCC
creates the .cfi
directives and the Assembler puts them into the .eh_frame
section.
An example of gcc -S
:
.file "unwind.c"
.section .text
.align 4
.type unwind_stop, @function
unwind_stop:
.LFB83:
.cfi_startproc
l.addi r1, r1, -28
.cfi_def_cfa_offset 28
l.sw 0(r1), r16
l.sw 4(r1), r18
l.sw 8(r1), r20
l.sw 12(r1), r22
l.sw 16(r1), r24
l.sw 20(r1), r26
l.sw 24(r1), r9
.cfi_offset 16, -28
.cfi_offset 18, -24
.cfi_offset 20, -20
.cfi_offset 22, -16
.cfi_offset 24, -12
.cfi_offset 26, -8
.cfi_offset 9, -4
l.or r24, r8, r8
l.or r22, r10, r10
l.lwz r18, -1172(r10)
l.lwz r20, -692(r10)
l.lwz r17, -688(r10)
l.add r20, r20, r17
l.andi r16, r4, 16
l.sfnei r16, 0
When looking at the glibc build I noticed the .eh_frame
data for
__futex_abstimed_wait_cancelable64
is missing from futex-internal.o. The one
where unwinding is failing we find it was completely mising .cfi
directives.
Why is GCC not generating .cfi
directives for this file?
.file "futex-internal.c"
.section .text
.section .rodata.str1.1,"aMS",@progbits,1
.LC0:
.string "The futex facility returned an unexpected error code.\n"
.section .text
.align 4
.global __futex_abstimed_wait_cancelable64
.type __futex_abstimed_wait_cancelable64, @function
__futex_abstimed_wait_cancelable64:
l.addi r1, r1, -20
l.sw 0(r1), r16
l.sw 4(r1), r18
l.sw 8(r1), r20
l.sw 12(r1), r22
l.sw 16(r1), r9
l.or r22, r3, r3
l.or r20, r4, r4
l.or r16, r6, r6
l.sfnei r6, 0
l.ori r17, r0, 1
l.cmov r17, r17, r0
l.sfeqi r17, 0
l.bnf .L14
l.nop
Looking closer at the build line of these 2 files I see the build of futex-internal.c
is missing -fexceptions
.
This flag is needed to enable the eh_frame
section, which is what powers C++
exceptions, the flag is needed when we are building C code which needs to
support C++ exceptions.
So why is it not enabled? Is this a problem with the GLIBC build?
Looking at GLIBC the nptl/Makefile
set’s -fexceptions
explicitly for each
c file that needs it. For example:
# The following are cancellation points. Some of the functions can
# block and therefore temporarily enable asynchronous cancellation.
# Those must be compiled asynchronous unwind tables.
CFLAGS-pthread_testcancel.c += -fexceptions
CFLAGS-pthread_join.c += -fexceptions -fasynchronous-unwind-tables
CFLAGS-pthread_timedjoin.c += -fexceptions -fasynchronous-unwind-tables
CFLAGS-pthread_clockjoin.c += -fexceptions -fasynchronous-unwind-tables
CFLAGS-pthread_once.c += $(uses-callbacks) -fexceptions \
-fasynchronous-unwind-tables
CFLAGS-pthread_cond_wait.c += -fexceptions -fasynchronous-unwind-tables
CFLAGS-sem_wait.c += -fexceptions -fasynchronous-unwind-tables
CFLAGS-sem_timedwait.c += -fexceptions -fasynchronous-unwind-tables
CFLAGS-sem_clockwait.c = -fexceptions -fasynchronous-unwind-tables
It is missing such a line for futex-internal.c
. The following patch and a
libpthread rebuild fixes the issue!
--- a/nptl/Makefile
+++ b/nptl/Makefile
@@ -220,6 +220,7 @@ CFLAGS-pthread_cond_wait.c += -fexceptions -fasynchronous-unwind-tables
CFLAGS-sem_wait.c += -fexceptions -fasynchronous-unwind-tables
CFLAGS-sem_timedwait.c += -fexceptions -fasynchronous-unwind-tables
CFLAGS-sem_clockwait.c = -fexceptions -fasynchronous-unwind-tables
+CFLAGS-futex-internal.c += -fexceptions -fasynchronous-unwind-tables
# These are the function wrappers we have to duplicate here.
CFLAGS-fcntl.c += -fexceptions -fasynchronous-unwind-tables
I submitted this patch
to GLIBC but it turns out it was already fixed upstream
a few weeks before. Doh.
Summary
I hope the investigation into debugging this C++ exception test case proved interesting.
We can learn a lot about the deep internals of our tools when we have to fix bugs in them.
Like most illusive bugs, in the end this was a trivial fix but required some
key background knowledge.
Additional Reading
21 Jul 2020
This is an ongoing series of posts on ELF Binary Relocations and Thread
Local Storage. This article covers only Thread Local Storage and assumes
the reader has had a primer in ELF Relocations, if not please start with
my previous article ELF Binaries and Relocation Entries.
This is the third part in an illustrated 3 part series covering:
In the last article we covered how Thread Local Storage (TLS) works at runtime,
but how do we get there? How does the compiler and linker create the memory
structures and code fragments described in the previous article?
In this article we will discuss how TLS relocations are is implemented. Our
outline:
As before, the examples in this article can be found in my tls-examples
project. Please check it out.
I will assume here that most people understand what a compiler and assembler
basically do. In the sense that compiler will compile routines
written C code or something similar to assembly language. It is then up to the
assembler to turn that assembly code into machine code to run on a CPU.
That is a big part of what a toolchain does, and it’s pretty much that simple if
we have a single file of source code. But usually we don’t have a single file,
we have the multiple files, the c runtime,
crt0 and other libraries like
libc. These all need to be
put together into our final program, that is where the complexities of the
linker comes in.
In this article I will cover how variables in our source code (symbols) traverse
the toolchain from code to the memory in our final running program. A picture that looks
something like this:
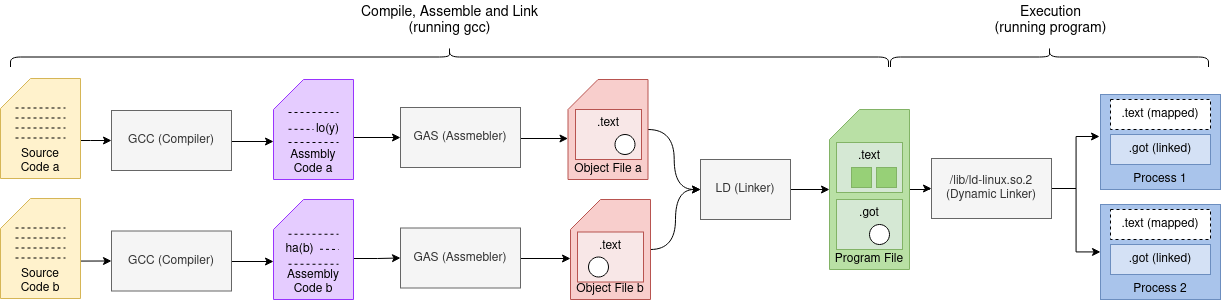
The Compiler
First we start off with how relocations are created and emitted in the compiler.
As I work primarily on the GNU toolchain with
it’s GCC compiler we will look at that, let’s get started.
GCC Legitimize Address
To start we define a symbol as named address in memory. This address can be
a program variable where data is stored or function reference to where a
subroutine starts.
In GCC we have have TARGET_LEGITIMIZE_ADDRESS
, the OpenRISC implementation
being or1k_legitimize_address().
It takes a symbol (memory address) and makes it usable in our CPU by generating RTX
sequences that are possible on our CPU to load that address into a register.
RTX represents a tree node in GCC’s register transfer language (RTL). The RTL
Expression is used to express our algorithm as a series of register transfers.
This is used as register transfer is basically what a CPU does.
A snippet from legitimize_address()
function is below. The argument x
represents our input symbol (memory address) that we need to make usable by our
CPU. This code uses GCC internal API’s to emit RTX code sequences.
static rtx
or1k_legitimize_address (rtx x, rtx /* unused */, machine_mode /* unused */)
...
case TLS_MODEL_NONE:
t1 = can_create_pseudo_p () ? gen_reg_rtx (Pmode) : scratch;
if (!flag_pic)
{
emit_insn (gen_rtx_SET (t1, gen_rtx_HIGH (Pmode, x)));
return gen_rtx_LO_SUM (Pmode, t1, x);
}
else if (is_local)
{
crtl->uses_pic_offset_table = 1;
t2 = gen_sym_unspec (x, UNSPEC_GOTOFF);
emit_insn (gen_rtx_SET (t1, gen_rtx_HIGH (Pmode, t2)));
emit_insn (gen_add3_insn (t1, t1, pic_offset_table_rtx));
return gen_rtx_LO_SUM (Pmode, t1, copy_rtx (t2));
}
else
{
...
We can read the code snippet above as follows:
- This is for the non
TLS
case as we see TLS_MODEL_NONE
.
- We reserve a temporary register
t1
.
- If not using Position-independent code (
flag_pic
) we do:
- Emit an instruction to put the high bits of
x
into our temporary register t1
.
- Return the sum of
t1
and the low bits of x
.
- Otherwise if the symbol is static (
is_local
) we do:
- Mark the global state that this object file uses the
uses_pic_offset_table
.
- We create a Global Offset Table offset variable
t2
.
- Emit an instruction to put the high bits of
t2
(the GOT offset) into out temporary register t1
.
- Emit an instruction to put the sum of
t1
(high bits of t2) and the GOT into
t1`.
- Return the sum of
t1
and the low bits of t1
.
You may have noticed that the local symbol still used the global offset
table (GOT). This is
because Position-idependent code requires using the GOT to reference symbols.
An example, from nontls.c:
static int x;
int *get_x_addr() {
return &x;
}
Example of the non pic case above, when we look at the assembly code generated by GCC
we can see the following:
.file "nontls.c"
.section .text
.local x
.comm x,4,4
.align 4
.global get_x_addr
.type get_x_addr, @function
get_x_addr:
l.addi r1, r1, -8 # \
l.sw 0(r1), r2 # | function prologue
l.addi r2, r1, 8 # |
l.sw 4(r1), r9 # /
l.movhi r17, ha(x) # \__ legitimize address of x into r17
l.addi r17, r17, lo(x) # /
l.or r11, r17, r17 # } place result in return register r11
l.lwz r2, 0(r1) # \
l.lwz r9, 4(r1) # | function epilogue
l.addi r1, r1, 8 # |
l.jr r9 # |
l.nop # /
.size get_x_addr, .-get_x_addr
.ident "GCC: (GNU) 9.0.1 20190409 (experimental)"
Example of the local pic case above the same code compiled with the -fPIC
GCC option
looks like the following:
.file "nontls.c"
.section .text
.local x
.comm x,4,4
.align 4
.global get_x_addr
.type get_x_addr, @function
get_x_addr:
l.addi r1, r1, -8 # \
l.sw 0(r1), r2 # | function prologue
l.addi r2, r1, 8 # |
l.sw 4(r1), r9 # /
l.jal 8 # \
l.movhi r19, gotpchi(_GLOBAL_OFFSET_TABLE_-4) # | PC relative, put
l.ori r19, r19, gotpclo(_GLOBAL_OFFSET_TABLE_+0) # | GOT into r19
l.add r19, r19, r9 # /
l.movhi r17, gotoffha(x) # \
l.add r17, r17, r19 # | legitimize address of x into r17
l.addi r17, r17, gotofflo(x) # /
l.or r11, r17, r17 # } place result in return register r11
l.lwz r2, 0(r1) # \
l.lwz r9, 4(r1) # | function epilogue
l.addi r1, r1, 8 # |
l.jr r9 # |
l.nop # /
.size get_x_addr, .-get_x_addr
.ident "GCC: (GNU) 9.0.1 20190409 (experimental)"
TLS and Addend cases are also handled by or1k_legitimize_address()
.
GCC Print Operand
Once RTX is generated by legitimize address and GCC passes
run all of their optimizations the RTX needs to be printed out as assembly code. During
this process relocations are printed by GCC macros TARGET_PRINT_OPERAND_ADDRESS
and TARGET_PRINT_OPERAND
. In OpenRISC these defined
by or1k_print_operand_address()
and or1k_print_operand().
Let us have a look at or1k_print_operand_address()
.
/* Worker for TARGET_PRINT_OPERAND_ADDRESS.
Prints the argument ADDR, an address RTX, to the file FILE. The output is
formed as expected by the OpenRISC assembler. Examples:
RTX OUTPUT
(reg:SI 3) 0(r3)
(plus:SI (reg:SI 3) (const_int 4)) 0x4(r3)
(lo_sum:SI (reg:SI 3) (symbol_ref:SI ("x"))) lo(x)(r3) */
static void
or1k_print_operand_address (FILE *file, machine_mode, rtx addr)
{
rtx offset;
switch (GET_CODE (addr))
{
case REG:
fputc ('0', file);
break;
case ...
case LO_SUM:
offset = XEXP (addr, 1);
addr = XEXP (addr, 0);
print_reloc (file, offset, 0, RKIND_LO);
break;
default: ...
}
fprintf (file, "(%s)", reg_names[REGNO (addr)]);
}
The above code snippet can be read as we explain below, but let’s first
make some notes:
- The input RTX
addr
for TARGET_PRINT_OPERAND_ADDRESS
will usually contain
a register and an offset typically this is used for LOAD and STORE
operations.
- Think of the RTX
addr
as a node in an AST.
- The RTX node with code
REG
and SYMBOL_REF
are always leaf nodes.
With that, and if we use the or1k_print_operand_address()
c comments above as examples
of some RTX addr
input we will have:
RTX | (reg:SI 3) (lo_sum:SI (reg:SI 3) (symbol_ref:SI("x")))
-----------+--------------------------------------------------------------------
TREE |
(code) | (code:REG regno:3) (code:LO_SUM)
/ \ | / \
(0) (1) | (code:REG regno:3) (code:SYMBOL_REF "x")
We can now read the above snippet as:
- First get the
CODE
of the RTX.
- If
CODE
is REG
(a register) than our offset can be 0
.
- If
IS
is LO_SUM
(an addition operation) then we need to break it down to:
- Arg
0
is our new addr
RTX (which we assume is a register)
- Arg
1
is an offset (which we then print with print_reloc())
- Second print out the register name now in
addr
i.e. “r3”.
The code of or1k_print_operand()
is similar and the reader may be inclined to
read more details. With that we can move on to the assembler.
TLS cases are also handled inside of the print_reloc()
function.
The Assembler
In the GNU Toolchain our assembler is GAS, part of binutils.
The code that handles relocations is found in the function
parse_reloc()
found in opcodes/or1k-asm.c
. The function parse_reloc()
is the direct counterpart of GCC’s print_reloc()
discussed above. This is actually part of or1k_cgen_parse_operand()
which is wired into our assembler generator CGEN used for parsing operands.
If we are parsing a relocation like the one from above lo(x)
then we can
isolate the code that processes that relocation.
static const bfd_reloc_code_real_type or1k_imm16_relocs[][6] = {
{ BFD_RELOC_LO16,
BFD_RELOC_OR1K_SLO16,
...
BFD_RELOC_OR1K_TLS_LE_AHI16 },
};
static int
parse_reloc (const char **strp)
{
const char *str = *strp;
enum or1k_rclass cls = RCLASS_DIRECT;
enum or1k_rtype typ;
...
else if (strncasecmp (str, "lo(", 3) == 0)
{
str += 3;
typ = RTYPE_LO;
}
...
*strp = str;
return (cls << RCLASS_SHIFT) | typ;
}
This uses strncasecmp to match
our "lo("
string pattern. The returned result is a relocation type and relocation class
which are use to lookup the relocation BFD_RELOC_LO16
in the or1k_imm16_relocs[][]
table
which is indexed by relocation class and relocation class.
The assembler will encode that into the ELF binary. For TLS relocations the exact same
pattern is used.
The Linker
In the GNU Toolchain our object linker is the GNU linker LD, also part of the
binutils project.
The GNU linker uses the framework
BFD or Binary File
Descriptor which
is a beast. It is not only used in the linker but also used in GDB, the GNU
Simulator and the objdump tool.
What makes this possible is a rather complex API.
BFD Linker API
The BFD API is a generic binary file access API. It has been designed to support multiple
file formats and architectures via an object oriented, polymorphic API all written in c. It supports file formats
including a.out,
COFF and
ELF as well as
unexpected file formats like
verilog hex memory dumps.
Here we will concentrate on the BFD ELF implementation.
The API definition is split across multiple files which include:
- bfd/bfd-in.h - top level generic APIs including
bfd_hash_table
- bfd/bfd-in2.h - top level binary file APIs including
bfd
and asection
- include/bfdlink.h - generic bfd linker APIs including
bfd_link_info
and bfd_link_hash_table
- bfd/elf-bfd.h - extensions to the APIs for ELF binaries including
elf_link_hash_table
bfd/elf{wordsize}-{architecture}.c
- architecture specific implementations
For each architecture implementations are defined in bfd/elf{wordsize}-{architecture}.c
. For
example for OpenRISC we have
bfd/elf32-or1k.c.
Throughout the linker code we see access to the BFD Linker and ELF APIs. Some key symbols to watch out for include:
info
- A reference to bfd_link_info
top level reference to all linker
state.
htab
- A pointer to elf_or1k_link_hash_table
from or1k_elf_hash_table (info)
, a hash
table on steroids which stores generic link state and arch specific state, it’s also a hash
table of all global symbols by name, contains:
htab->root.splt
- the output .plt
section
htab->root.sgot
- the output .got
section
htab->root.srelgot
- the output .relgot
section (relocations against the got)
htab->root.sgotplt
- the output .gotplt
section
htab->root.dynobj
- a special bfd
to which sections are added (created in or1k_elf_check_relocs
)
sym_hashes
- From elf_sym_hashes (abfd)
a list of for global symbols
in a bfd
indexed by the relocation index ELF32_R_SYM (rel->r_info)
.
h
- A pointer to a struct elf_link_hash_entry
, represents link state
of a global symbol, contains:
h->got
- A union of different attributes with different roles based on link phase.
h->got.refcount
- used during phase 1 to count the symbol .got
section references
h->got.offset
- used during phase 2 to record the symbol .got
section offset
h->plt
- A union with the same function as h->got
but used for the .plt
section.
h->root.root.string
- The symbol name
local_got
- an array of unsigned long
from elf_local_got_refcounts (ibfd)
with the same
function to h->got
but for local symbols, the function of the unsigned long
is changed base
on the link phase. Ideally this should also be a union.
tls_type
- Retrieved by ((struct elf_or1k_link_hash_entry *) h)->tls_type
used to store the
tls_type
of a global symbol.
local_tls_type
- Retrieved by elf_or1k_local_tls_type(abfd)
entry to store tls_type
for local
symbols, when h
is NULL
.
root
- The struct field root
is used in subclasses to represent the parent class, similar to how super
is used
in other languages.
Putting it all together we have a diagram like the following:
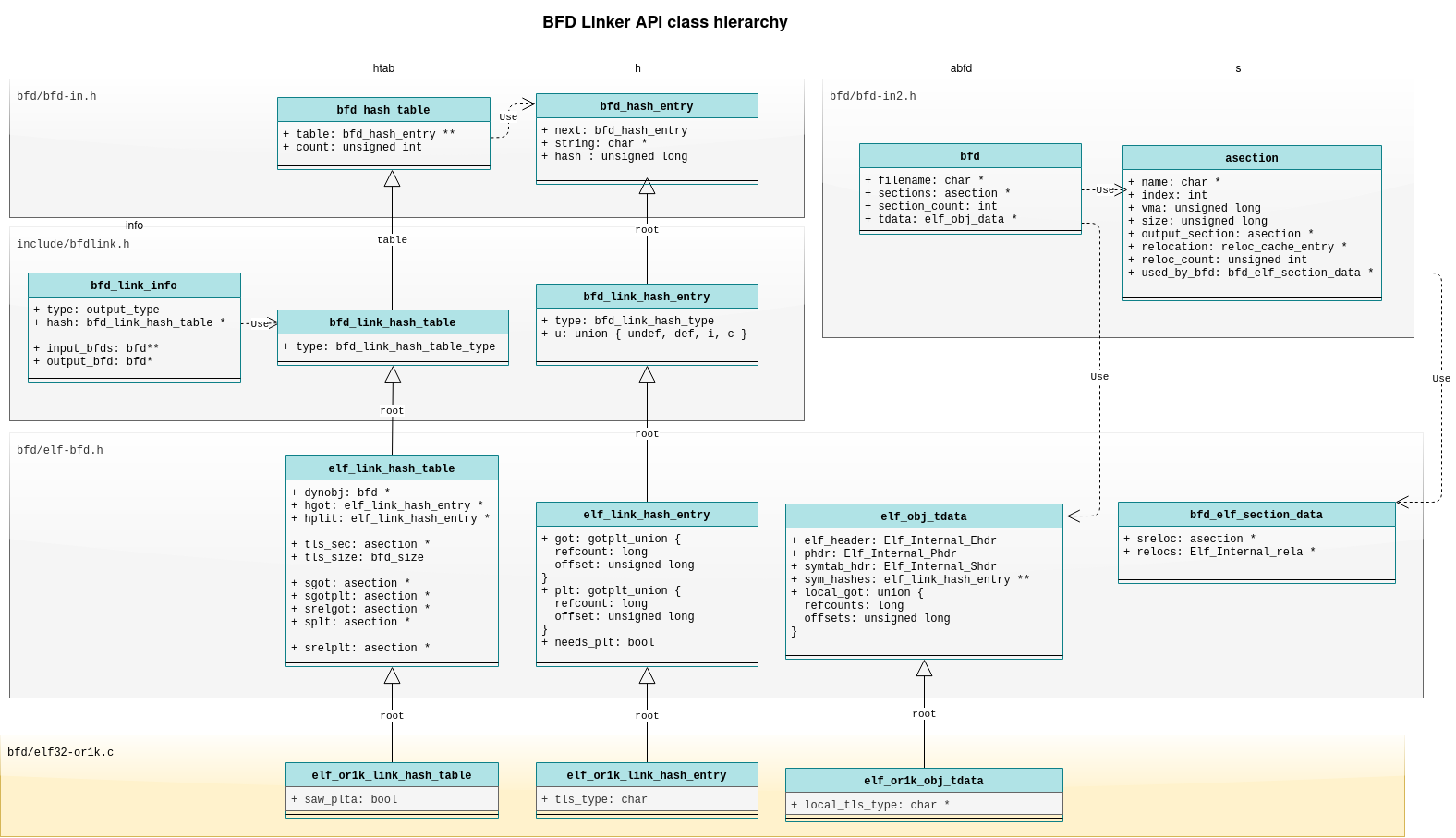
Now that we have a bit of understanding of the data structures
we can look to the link algorithm.
The link process in the GNU Linker can be thought of in phases.
Phase 1 - Book Keeping (check_relocs)
The or1k_elf_check_relocs()
function is called during the first phase to
do book keeping on relocations. The function signature looks like:
static bfd_boolean
or1k_elf_check_relocs (bfd *abfd,
struct bfd_link_info *info,
asection *sec,
const Elf_Internal_Rela *relocs)
#define elf_backend_check_relocs or1k_elf_check_relocs
The arguments being:
abfd
- The current elf object file we are working on
info
- The BFD API
sec
- The current elf section we are working on
relocs
- The relocations from the current section
It does the book keeping by looping over relocations for the provided section
and updating the local and global symbol properties.
For local symbols:
...
else
{
unsigned char *local_tls_type;
/* This is a TLS type record for a local symbol. */
local_tls_type = (unsigned char *) elf_or1k_local_tls_type (abfd);
if (local_tls_type == NULL)
{
bfd_size_type size;
size = symtab_hdr->sh_info;
local_tls_type = bfd_zalloc (abfd, size);
if (local_tls_type == NULL)
return FALSE;
elf_or1k_local_tls_type (abfd) = local_tls_type;
}
local_tls_type[r_symndx] |= tls_type;
}
...
else
{
bfd_signed_vma *local_got_refcounts;
/* This is a global offset table entry for a local symbol. */
local_got_refcounts = elf_local_got_refcounts (abfd);
if (local_got_refcounts == NULL)
{
bfd_size_type size;
size = symtab_hdr->sh_info;
size *= sizeof (bfd_signed_vma);
local_got_refcounts = bfd_zalloc (abfd, size);
if (local_got_refcounts == NULL)
return FALSE;
elf_local_got_refcounts (abfd) = local_got_refcounts;
}
local_got_refcounts[r_symndx] += 1;
}
The above is pretty straight forward and we can read as:
- First part is for storing local symbol
TLS
type information:
- If the
local_tls_type
array is not initialized:
- Allocate it, 1 entry for each local variable
- Record the TLS type in
local_tls_type
for the current symbol
- Second part is for recording
.got
section references:
- If the
local_got_refcounts
array is not initialized:
- Allocate it, 1 entry for each local variable
- Record a reference by incrementing
local_got_refcounts
for the current symbol
For global symbols, it’s much more easy we see:
...
if (h != NULL)
((struct elf_or1k_link_hash_entry *) h)->tls_type |= tls_type;
else
...
if (h != NULL)
h->got.refcount += 1;
else
...
As the tls_type
and refcount
fields are available directly on each
hash_entry
handling global symbols is much easier.
- First part is for storing
TLS
type information:
- Record the TLS type in
tls_type
for the current hash_entry
- Second part is for recording
.got
section references:
- Record a reference by incrementing
got.refcounts
for the hash_entry
The above is repeated for all relocations and all input sections. A few other
things are also done including accounting for .plt
entries.
Phase 2 - creating space (size_dynamic_sections + _bfd_elf_create_dynamic_sections)
The or1k_elf_size_dynamic_sections()
function iterates over all input object files to calculate the size required for
output sections. The _bfd_elf_create_dynamic_sections()
function does the
actual section allocation, we use the generic version.
Setting up the sizes of the .got
section (global offset table) and .plt
section (procedure link table) is done here.
The definition is as below:
static bfd_boolean
or1k_elf_size_dynamic_sections (bfd *output_bfd ATTRIBUTE_UNUSED,
struct bfd_link_info *info)
#define elf_backend_size_dynamic_sections or1k_elf_size_dynamic_sections
#define elf_backend_create_dynamic_sections _bfd_elf_create_dynamic_sections
The arguments to or1k_elf_size_dynamic_sections()
being:
output_bfd
- Unused, the output elf object
info
- the BFD API which provides access to everything we need
Internally the function uses:
htab
- from or1k_elf_hash_table (info)
htab->root.dynamic_sections_created
- true
if sections like .interp
have been created by the linker
ibfd
- a bfd
pointer from info->input_bfds
, represents an input object when iterating.
s->size
- represents the output .got
section size, which we will be
incrementing.
srel->size
- represents the output .got.rela
section size, which will
contain relocations against the .got
section
During the first part of phase 2 we set .got
and .got.rela
section sizes
for local symbols with this code:
/* Set up .got offsets for local syms, and space for local dynamic
relocs. */
for (ibfd = info->input_bfds; ibfd != NULL; ibfd = ibfd->link.next)
{
...
local_got = elf_local_got_refcounts (ibfd);
if (!local_got)
continue;
symtab_hdr = &elf_tdata (ibfd)->symtab_hdr;
locsymcount = symtab_hdr->sh_info;
end_local_got = local_got + locsymcount;
s = htab->root.sgot;
srel = htab->root.srelgot;
local_tls_type = (unsigned char *) elf_or1k_local_tls_type (ibfd);
for (; local_got < end_local_got; ++local_got)
{
if (*local_got > 0)
{
unsigned char tls_type = (local_tls_type == NULL)
? TLS_UNKNOWN
: *local_tls_type;
*local_got = s->size;
or1k_set_got_and_rela_sizes (tls_type, bfd_link_pic (info),
&s->size, &srel->size);
}
else
*local_got = (bfd_vma) -1;
if (local_tls_type)
++local_tls_type;
}
}
Here, for example, we can see we iterate over each input elf object ibfd
and
each local symbol (local_got
) we try and update s->size
and srel->size
to
account for the required size.
The above can be read as:
- For each
local_got
entry:
- If the local symbol is used in the
.got
section:
- Get the
tls_type
byte stored in the local_tls_type
array
- Set the offset
local_got
to the section offset s->size
, that is used
in phase 3 to tell us where we need to write the symbol into the .got
section.
- Update
s->size
and srel->size
using or1k_set_got_and_rela_sizes()
- If the local symbol is not used in the
.got
section:
- Set the offset
local_got
to the -1
, to indicate not used
In the next part of phase 2 we allocate space for all global symbols by
iterating through symbols in htab
with the allocate_dynrelocs
iterator. To
do that we call:
elf_link_hash_traverse (&htab->root, allocate_dynrelocs, info);
Inside allocate_dynrelocs()
we record the space used for relocations and
the .got
and .plt
sections. Example:
if (h->got.refcount > 0)
{
asection *sgot;
bfd_boolean dyn;
unsigned char tls_type;
...
sgot = htab->root.sgot;
h->got.offset = sgot->size;
tls_type = ((struct elf_or1k_link_hash_entry *) h)->tls_type;
dyn = htab->root.dynamic_sections_created;
dyn = WILL_CALL_FINISH_DYNAMIC_SYMBOL (dyn, bfd_link_pic (info), h);
or1k_set_got_and_rela_sizes (tls_type, dyn,
&sgot->size, &htab->root.srelgot->size);
}
else
h->got.offset = (bfd_vma) -1;
The above, with h
being our global symbol, a pointer to struct elf_link_hash_entry
,
can be read as:
- If the symbol will be in the
.got
section:
- Get the global reference to the
.got
section and put it in sgot
- Set the got location
h->got.offset
for the symbol to the current got
section size htab->root.sgot
.
- Set
dyn
to true
if we will be doing a dynamic link.
- Call
or1k_set_got_and_rela_sizes()
to update the sizes for the .got
and .got.rela
sections.
- If the symbol is going to be in the
.got
section:
- Set the got location
h->got.offset
to -1
The function or1k_set_got_and_rela_sizes()
used above is used to increment
.got
and .rela
section sizes accounting for if these are TLS symbols, which
need additional entries and relocations.
Phase 3 - linking (relocate_section)
The or1k_elf_relocate_section()
function is called to fill in the relocation holes in the output binary .text
section. It does this by looping over relocations and writing to the .text
section the correct symbol value (memory address). It also updates other output
binary sections like the .got
section. Also, for dynamic executables and
libraries new relocations may be written to .rela
sections.
The function signature looks as follows:
static bfd_boolean
or1k_elf_relocate_section (bfd *output_bfd,
struct bfd_link_info *info,
bfd *input_bfd,
asection *input_section,
bfd_byte *contents,
Elf_Internal_Rela *relocs,
Elf_Internal_Sym *local_syms,
asection **local_sections)
#define elf_backend_relocate_section or1k_elf_relocate_section
The arguments to or1k_elf_relocate_sectioni()
being:
output_bfd
- the output elf object we will be writing to
info
- the BFD API which provides access to everything we need
input_bfd
- the current input elf object being iterated over
input_section
the current .text
section in the input elf object being iterated
over. From here we get .text
section output details for pc relative relocations:
input_section->output_section->vma
- the location of the output section.
input_section->output_offset
- the output offset
contents
- the output file buffer we will write to
relocs
- relocations from the current input section
local_syms
- an array of local symbols used to get the relocation
value for local symbols
local_sections
- an array input sections for local symbols, used to get the relocation
value for local symbols
Internally the function uses:
- or1k_elf_howto_table - not
mentioned until now, but an array of
howto
structs indexed by relocation enum.
The howto
struct expresses the algorithm required to update the relocation.
relocation
- a bfd_vma
the value of the relocation symbol (memory address)
to be written to the output file.
in the output file that needs to be updated for the relocation.
value
- the value that needs to be written to the relocation location.
During the first part of relocate_section
we see:
if (r_symndx < symtab_hdr->sh_info)
{
sym = local_syms + r_symndx;
sec = local_sections[r_symndx];
relocation = _bfd_elf_rela_local_sym (output_bfd, sym, &sec, rel);
name = bfd_elf_string_from_elf_section
(input_bfd, symtab_hdr->sh_link, sym->st_name);
name = name == NULL ? bfd_section_name (sec) : name;
}
else
{
bfd_boolean unresolved_reloc, warned, ignored;
RELOC_FOR_GLOBAL_SYMBOL (info, input_bfd, input_section, rel,
r_symndx, symtab_hdr, sym_hashes,
h, sec, relocation,
unresolved_reloc, warned, ignored);
name = h->root.root.string;
}
This can be read as:
- If the current symbol is a local symbol:
- We initialize
relocation
to the local symbol value using _bfd_elf_rela_local_sym()
.
- Otherwise the current symbol is global:
- We use the
RELOC_FOR_GLOBAL_SYMBOL()
macro to initialize relocation
.
During the next part we use the howto
information to update the relocation
value, and also
add relocations to the output file. For example:
case R_OR1K_TLS_GD_HI16:
case R_OR1K_TLS_GD_LO16:
case R_OR1K_TLS_GD_PG21:
case R_OR1K_TLS_GD_LO13:
case R_OR1K_TLS_IE_HI16:
case R_OR1K_TLS_IE_LO16:
case R_OR1K_TLS_IE_PG21:
case R_OR1K_TLS_IE_LO13:
case R_OR1K_TLS_IE_AHI16:
{
bfd_vma gotoff;
Elf_Internal_Rela rela;
asection *srelgot;
bfd_byte *loc;
bfd_boolean dynamic;
int indx = 0;
unsigned char tls_type;
srelgot = htab->root.srelgot;
/* Mark as TLS related GOT entry by setting
bit 2 to indcate TLS and bit 1 to indicate GOT. */
if (h != NULL)
{
gotoff = h->got.offset;
tls_type = ((struct elf_or1k_link_hash_entry *) h)->tls_type;
h->got.offset |= 3;
}
else
{
unsigned char *local_tls_type;
gotoff = local_got_offsets[r_symndx];
local_tls_type = (unsigned char *) elf_or1k_local_tls_type (input_bfd);
tls_type = local_tls_type == NULL ? TLS_NONE
: local_tls_type[r_symndx];
local_got_offsets[r_symndx] |= 3;
}
/* Only process the relocation once. */
if ((gotoff & 1) != 0)
{
gotoff += or1k_initial_exec_offset (howto, tls_type);
/* The PG21 and LO13 relocs are pc-relative, while the
rest are GOT relative. */
relocation = got_base + (gotoff & ~3);
if (!(r_type == R_OR1K_TLS_GD_PG21
|| r_type == R_OR1K_TLS_GD_LO13
|| r_type == R_OR1K_TLS_IE_PG21
|| r_type == R_OR1K_TLS_IE_LO13))
relocation -= got_sym_value;
break;
}
...
/* Static GD. */
else if ((tls_type & TLS_GD) != 0)
{
bfd_put_32 (output_bfd, 1, sgot->contents + gotoff);
bfd_put_32 (output_bfd, tpoff (info, relocation, dynamic),
sgot->contents + gotoff + 4);
}
gotoff += or1k_initial_exec_offset (howto, tls_type);
...
/* Static IE. */
else if ((tls_type & TLS_IE) != 0)
bfd_put_32 (output_bfd, tpoff (info, relocation, dynamic),
sgot->contents + gotoff);
/* The PG21 and LO13 relocs are pc-relative, while the
rest are GOT relative. */
relocation = got_base + gotoff;
if (!(r_type == R_OR1K_TLS_GD_PG21
|| r_type == R_OR1K_TLS_GD_LO13
|| r_type == R_OR1K_TLS_IE_PG21
|| r_type == R_OR1K_TLS_IE_LO13))
relocation -= got_sym_value;
}
break;
Here we process the relocation for TLS General Dynamic and Initial Exec relocations. I have trimmed
out the shared cases to save space.
This can be read as:
- Get a reference to the output relocation section
sreloc
.
- Get the got offset which we setup during phase 3 for global or local symbols.
- Mark the symbol as using a TLS got entry, this
offset |= 3
trick is
possible because on 32-bit machines we have 2 lower bits free. This
is used during phase 4.
- If we have already processed this symbol once:
- Update
relocation
to the location in the output .got
section and break, we only need to create .got
entries 1 time
- Otherwise populate
.got
section entries
- For General Dynamic
- Put 2 entries into the output elf object
.got
section, a literal 1
and the thread pointer offset
- For Initial Exec
- Put 1 entry into the output elf object
.got
section, the thread pointer offset
- Finally update the
relocation
to the location in the output .got
section
In the last part of the loop we write the relocation
value to the output
.text
section. This is done with the or1k_final_link_relocate()
function.
r = or1k_final_link_relocate (howto, input_bfd, input_section, contents,
rel->r_offset, relocation + rel->r_addend);
With this the .text
section is complete.
Phase 4 - finishing up (finish_dynamic_symbol + finish_dynamic_sections)
During phase 3 above we wrote the .text
section out to file. During the
final finishing up phase we need to write the remaining sections. This
includes the .plt
section an more writes to the .got
section.
This also includes the .plt.rela
and .got.rela
sections which contain
dynamic relocation entries.
Writing of the data sections is handled by
or1k_elf_finish_dynamic_sections()
and writing of the relocation sections is handled by
or1k_elf_finish_dynamic_symbol(). These are defined as below.
static bfd_boolean
or1k_elf_finish_dynamic_sections (bfd *output_bfd,
struct bfd_link_info *info)
static bfd_boolean
or1k_elf_finish_dynamic_symbol (bfd *output_bfd,
struct bfd_link_info *info,
struct elf_link_hash_entry *h,
Elf_Internal_Sym *sym)
#define elf_backend_finish_dynamic_sections or1k_elf_finish_dynamic_sections
#define elf_backend_finish_dynamic_symbol or1k_elf_finish_dynamic_symbol
A snippet for the or1k_elf_finish_dynamic_sections()
shows how when writing to
the .plt
section assembly code needs to be injected. This is where the first
entry in the .plt
section is written.
else if (bfd_link_pic (info))
{
plt0 = OR1K_LWZ(15, 16) | 8; /* .got+8 */
plt1 = OR1K_LWZ(12, 16) | 4; /* .got+4 */
plt2 = OR1K_NOP;
}
else
{
unsigned ha = ((got_addr + 0x8000) >> 16) & 0xffff;
unsigned lo = got_addr & 0xffff;
plt0 = OR1K_MOVHI(12) | ha;
plt1 = OR1K_LWZ(15,12) | (lo + 8);
plt2 = OR1K_LWZ(12,12) | (lo + 4);
}
or1k_write_plt_entry (output_bfd, splt->contents,
plt0, plt1, plt2, OR1K_JR(15));
elf_section_data (splt->output_section)->this_hdr.sh_entsize = 4;
Here we see a write to output_bfd
, this represents the output object file
which we are writing to. The argument splt->contents
represents the object
file offset to write to for the .plt
section. Next we see the line
elf_section_data (splt->output_section)->this_hdr.sh_entsize = 4
this allows the linker to calculate the size of the section.
A snippet from the or1k_elf_finish_dynamic_symbol()
function shows where
we write out the code and dynamic relocation entries for each symbol to
the .plt
section.
splt = htab->root.splt;
sgot = htab->root.sgotplt;
srela = htab->root.srelplt;
...
else
{
unsigned ha = ((got_addr + 0x8000) >> 16) & 0xffff;
unsigned lo = got_addr & 0xffff;
plt0 = OR1K_MOVHI(12) | ha;
plt1 = OR1K_LWZ(12,12) | lo;
plt2 = OR1K_ORI0(11) | plt_reloc;
}
or1k_write_plt_entry (output_bfd, splt->contents + h->plt.offset,
plt0, plt1, plt2, OR1K_JR(12));
/* Fill in the entry in the global offset table. We initialize it to
point to the top of the plt. This is done to lazy lookup the actual
symbol as the first plt entry will be setup by libc to call the
runtime dynamic linker. */
bfd_put_32 (output_bfd, plt_base_addr, sgot->contents + got_offset);
/* Fill in the entry in the .rela.plt section. */
rela.r_offset = got_addr;
rela.r_info = ELF32_R_INFO (h->dynindx, R_OR1K_JMP_SLOT);
rela.r_addend = 0;
loc = srela->contents;
loc += plt_index * sizeof (Elf32_External_Rela);
bfd_elf32_swap_reloca_out (output_bfd, &rela, loc);
Here we can see we write 3 things to output_bfd
for the single .plt
entry.
We write:
- The assembly code to the
.plt
section.
- The
plt_base_addr
(the first entry in the .plt
for runtime lookup) to the .got
section.
- And finally a dynamic relocation for our symbol to the
.plt.rela
.
With that we have written all of the sections out to our final elf object, and it’s ready
to be used.
GLIBC Runtime Linker
The runtime linker, also referred to as the dynamic linker, will do the final
linking as we load our program and shared libraries into memory. It can process
a limited set of relocation entries that were setup above during phase 4 of
linking.
The runtime linker implementation is found mostly in the
elf/dl-*
GLIBC source files. Dynamic relocation processing is handled in by
the _dl_relocate_object()
function in the elf/dl-reloc.c
file. The back end macro used for relocation
ELF_DYNAMIC_RELOCATE
is defined across several files including elf/dynamic-link.h
and elf/do-rel.h
Architecture specific relocations are handled by the function elf_machine_rela()
, the implementation
for OpenRISC being in sysdeps/or1k/dl-machine.h.
In summary from top down:
- elf/rtld.c - implements
dl_main()
the top level entry for the dynamic linker.
- elf/dl-open.c - function
dl_open_worker()
calls _dl_relocate_object()
, you may also recognize this from dlopen(3).
- elf/dl-reloc.c - function
_dl_relocate_object
calls ELF_DYNAMIC_RELOCATE
elf/dynamic-link.h
- defined macro ELF_DYNAMIC_RELOCATE
calls elf_dynamic_do_Rel()
via several macros
elf/do-rel.h
- function elf_dynamic_do_Rel()
calls elf_machine_rela()
sysdeps/or1k/dl-machine.h
- architecture specific function elf_machine_rela()
implements dynamic relocation handling
It supports relocations for:
R_OR1K_NONE
- do nothing
R_OR1K_COPY
- used to copy initial values from shared objects to process memory.
R_OR1K_32
- a 32-bit
value
R_OR1K_GLOB_DAT
- aligned 32-bit
values for GOT
entries
R_OR1K_JMP_SLOT
- aligned 32-bit
values for PLT
entries
R_OR1K_TLS_DTPMOD/R_OR1K_TLS_DTPOFF
- for shared TLS GD GOT
entries
R_OR1K_TLS_TPOFF
- for shared TLS IE GOT
entries
A snippet of the OpenRISC implementation of elf_machine_rela()
can be seen
below. It is pretty straight forward.
/* Perform the relocation specified by RELOC and SYM (which is fully resolved).
MAP is the object containing the reloc. */
auto inline void
__attribute ((always_inline))
elf_machine_rela (struct link_map *map, const Elf32_Rela *reloc,
const Elf32_Sym *sym, const struct r_found_version *version,
void *const reloc_addr_arg, int skip_ifunc)
{
struct link_map *sym_map = RESOLVE_MAP (&sym, version, r_type);
Elf32_Addr value = SYMBOL_ADDRESS (sym_map, sym, true);
...
switch (r_type)
{
...
case R_OR1K_32:
/* Support relocations on mis-aligned offsets. */
value += reloc->r_addend;
memcpy (reloc_addr_arg, &value, 4);
break;
case R_OR1K_GLOB_DAT:
case R_OR1K_JMP_SLOT:
*reloc_addr = value + reloc->r_addend;
break;
...
}
}
Handling TLS
The complicated part of the runtime linker is how it handles TLS variables.
This is done in the following files and functions.
The reader can read through the initialization code which is pretty straight forward, except for the
macros. Like most GNU code the code relies heavily on untyped macros. These macros are defined
in the architecture specific implementation files. For OpenRISC this is:
From the previous article on TLS we have the
TLS data structure that looks as follows:
dtv[] [ dtv[0], dtv[1], dtv[2], .... ]
counter ^ | \
----/ / \________
/ V V
/------TCB-------\/----TLS[1]----\ /----TLS[2]----\
| pthread tcbhead | tbss tdata | | tbss tdata |
\----------------/\--------------/ \--------------/
^
|
TP-----/
The symbols and macros defined in sysdeps/or1k/nptl/tls.h
are:
__thread_self
- a symbol representing the current thread always
TLS_DTV_AT_TP
- used throughout the TLS code to adjust offsets
TLS_TCB_AT_TP
- used throughout the TLS code to adjust offsets
TLS_TCB_SIZE
- used during init_tls()
to allocate memory for TLS
TLS_PRE_TCB_SIZE
- used during init_tls()
to allocate space for the pthread
struct
INSTALL_DTV
- used during initialization to update a new dtv pointer into the given tcb
GET_DTV
- gets dtv via the provided tcb pointer
INSTALL_NEW_DTV
- used during resizing to update the dtv into the current runtime __thread_self
TLS_INIT_TP
- sets __thread_self
this is the final step in init_tls()
THREAD_DTV
- gets dtv via _thread_self
THREAD_SELF
- get the pthread pointer via __thread_self
Implementations for OpenRISC are:
register tcbhead_t *__thread_self __asm__("r10");
#define TLS_DTV_AT_TP 1
#define TLS_TCB_AT_TP 0
#define TLS_TCB_SIZE sizeof (tcbhead_t)
#define TLS_PRE_TCB_SIZE sizeof (struct pthread)
#define INSTALL_DTV(tcbp, dtvp) (((tcbhead_t *) (tcbp))->dtv = (dtvp) + 1)
#define GET_DTV(tcbp) (((tcbhead_t *) (tcbp))->dtv)
#define TLS_INIT_TP(tcbp) ({__thread_self = ((tcbhead_t *)tcbp + 1); NULL;})
#define THREAD_DTV() ((((tcbhead_t *)__thread_self)-1)->dtv)
#define INSTALL_NEW_DTV(dtv) (THREAD_DTV() = (dtv))
#define THREAD_SELF \
((struct pthread *) ((char *) __thread_self - TLS_INIT_TCB_SIZE \
- TLS_PRE_TCB_SIZE))
Summary
We have looked at how symbols move from the Compiler, to Assembler, to Linker to
Runtime linker.
This has ended up being a long article to explain a rather complicated subject.
Let’s hope it helps provide a good reference for others who want to work on the
GNU toolchain in the future.
Further Reading
- GCC Passes - My blog entry on GCC passes
- bfdint - The BFD developer’s manual
- ldint - The LD developer’s manual
- LD and BFD Gist - Dump of notes I collected while working on this article.